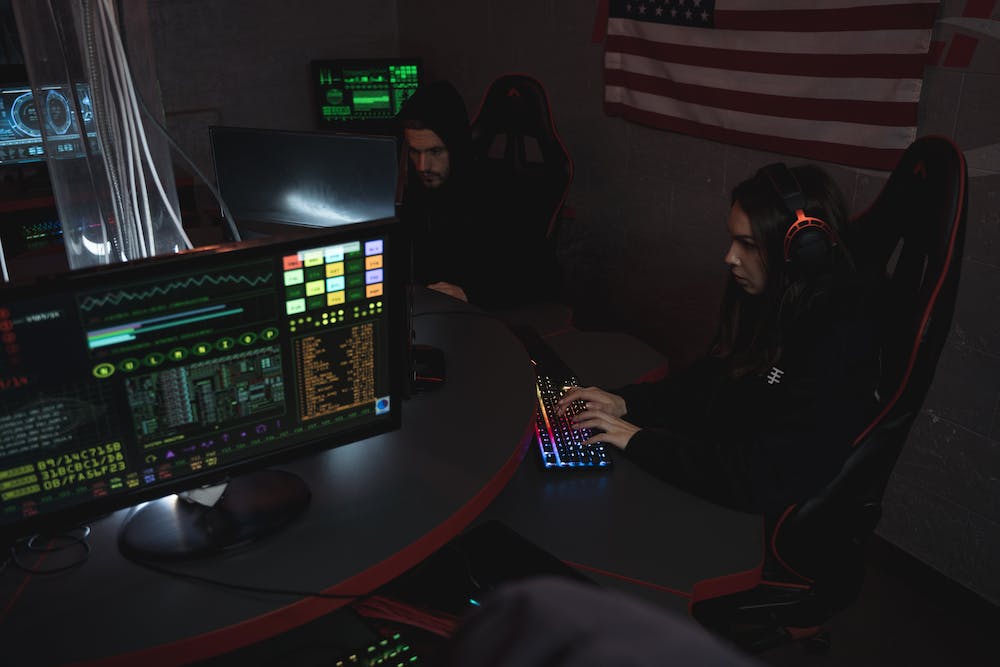
Laravel Debugbar is a powerful debugging tool that offers developers an easy and comprehensive way to debug their Laravel applications. With its intuitive interface and plethora of features, Debugbar makes finding and fixing bugs a breeze. In this guide, we will explore the various capabilities of Laravel Debugbar and learn how to use IT effectively for debugging our Laravel projects.
Installation
The first step to start using Laravel Debugbar is to install IT via Composer. Open your terminal, navigate to your Laravel project directory, and run the following command:
composer require barryvdh/laravel-debugbar --dev
Once the installation is complete, you will need to register the Debugbar service provider. Open the config/app.php
file and add the following line to the providers
array:
'providers' => [
// ...
Barryvdh\Debugbar\ServiceProvider::class,
],
Next, publish the configuration file by running the following command:
php artisan vendor:publish --provider="Barryvdh\Debugbar\ServiceProvider"
This will create a debugbar.php
configuration file in the config
directory. You can customize the Debugbar’s settings in this file, such as the enabled environment and excluded URLs.
Enabling the Debugbar
To enable the Debugbar, open the app/Providers/AppServiceProvider.php
file and add the following code to the register
method:
public function register()
{
if (config('app.debug')) {
$this->app->register(\Barryvdh\Debugbar\ServiceProvider::class);
}
}
This ensures that the Debugbar is only enabled when the application is in debug mode, as defined in the config/app.php
file. Restart your server, and the Debugbar should now be visible at the bottom of your Laravel application.
Using the Debugbar
The Debugbar provides a wealth of information and functionality for debugging your Laravel application. Let’s explore some of its key features:
Timing and Memory
The Debugbar shows the time and memory usage of each request, allowing you to identify any performance bottlenecks or memory leaks. IT provides a breakdown of the time spent on each part of your application, such as database queries, view rendering, and more.
Database Queries
The Debugbar captures all database queries performed during a request and displays them in a detailed and organized manner. You can see the SQL statement, bindings, execution time, and even analyze the query’s performance through the EXPLAIN feature.
Routes and Views
The Debugbar provides a convenient way to inspect the available routes and the views used to render the current request. You can see the route name, action, middleware, and even test the response directly from the Debugbar.
Logs and Messages
You can log custom messages to the Debugbar, making IT a powerful tool for debugging and monitoring your application. Additionally, Laravel’s default log messages are also displayed in the Debugbar, allowing you to quickly identify any errors or warnings.
Exceptions and Stack Trace
In case of exceptions, Laravel Debugbar captures the detailed stack trace and displays IT in an easy-to-read format. This helps you pinpoint the exact location and cause of the error, making the debugging process much smoother.
Custom Collectors and Extensions
The Debugbar allows you to create custom collectors and extensions to cater to your specific debugging needs. You can add additional data to the Debugbar or create entirely new tabs with custom functionality.
FAQs
Q: How can I disable the Debugbar in specific environments?
A: Open the config/debugbar.php
file and update the 'enabled'
key with the following code:
'enabled' => env('DEBUGBAR_ENABLED', false),
Then, in your environment-specific .env
file, add the following line to disable the Debugbar:
DEBUGBAR_ENABLED=false
Q: Can I share the Debugbar with specific users only?
A: Yes, you can restrict access to the Debugbar using the 'enabled'
key in the config/debugbar.php
file. You can customize the logic for enabling or disabling the Debugbar based on user roles or permissions.
Q: How can I add custom messages or log entries to the Debugbar?
A: You can log messages to the Debugbar using the debugbar
helper function provided by Laravel Debugbar. Simply call the function and pass in your message:
debugbar()->info('Custom message');
Q: Is Laravel Debugbar compatible with Laravel Vapor?
A: Yes, Laravel Debugbar can be used with Laravel Vapor. However, IT is recommended to disable the Debugbar in production environments to ensure optimal performance.
With Laravel Debugbar, debugging your Laravel applications becomes a seamless experience. Its extensive features and real-time insights enable you to squash bugs and optimize performance effectively. Start using Laravel Debugbar today and elevate your Laravel development workflow.