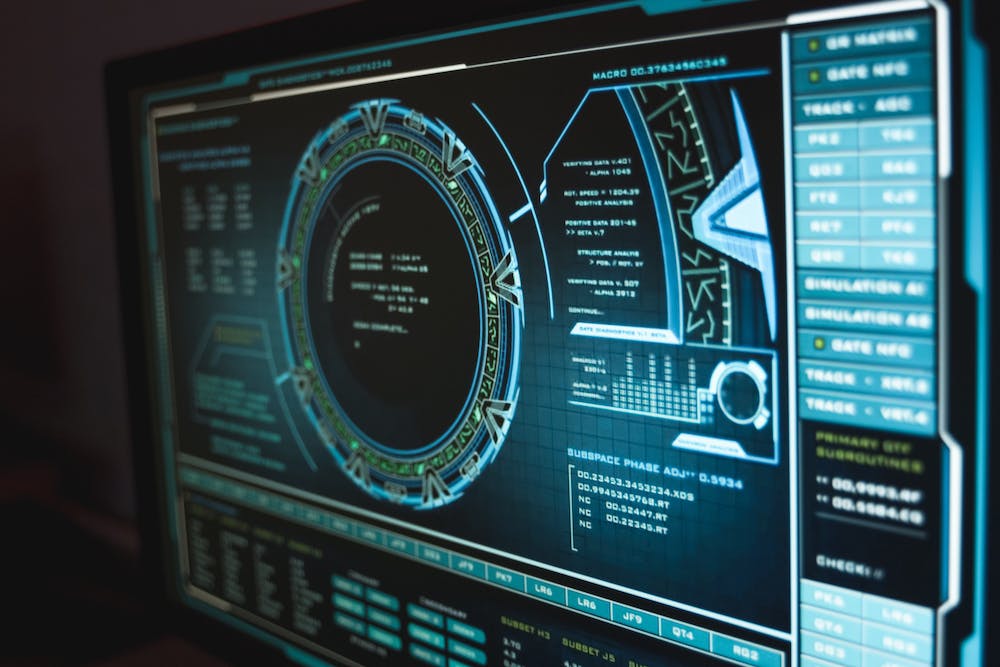
Laravel is a widely used PHP web framework that provides a convenient and secure way to authenticate users in web applications. Authentication is a crucial aspect of any web application as IT ensures that only authorized individuals can access certain features or resources. Laravel offers a variety of authentication features out of the box, making IT easy to implement robust user authentication in your projects. In this complete guide, we will explore Laravel’s authentication system in detail, covering everything from setting up authentication to customizing IT to suit your application’s needs.
Installation
Before diving into Laravel’s authentication system, you need to have Laravel installed on your system. If you haven’t already installed Laravel, you can do so by following the official documentation on Laravel’s Website. Once you have Laravel installed, you can start setting up authentication.
Laravel provides a convenient command-line tool, artisan
, to generate the scaffolding for authentication. Open your terminal or command prompt, navigate to your Laravel project’s directory, and run the following command:
php artisan make:auth
This command will generate the necessary controllers, views, and routes for authentication in your application. IT will also migrate the database to add the required tables for user authentication.
Authentication Routes
Laravel’s authentication system provides several routes out of the box to handle user registration, login, and logout. These routes are defined in the routes/web.php
file. Here is a list of the default authentication routes:
Registration
Route::get('/register', 'RegisterController@showRegistrationForm')->name('register');
Route::post('/register', 'RegisterController@register');
Login
Route::get('/login', 'LoginController@showLoginForm')->name('login');
Route::post('/login', 'LoginController@login');
Route::post('/logout', 'LoginController@logout')->name('logout');
These routes handle displaying the registration and login forms, as well as submitting the form for registration and login. The last route logs the user out and redirects them to the login page.
Controllers and Views
When you run the make:auth
command, Laravel generates the necessary controllers and views for authentication. These controllers are responsible for handling user registration, login, and logout, while the views provide the HTML markup for the associated pages.
The generated controllers can be found in the app/Http/Controllers/Auth
directory. You can customize these controllers to add additional logic or modify the existing functionality as per your application’s requirements.
The corresponding views can be found in the resources/views/auth
directory. These views define the HTML markup for the registration and login forms, as well as other pages related to authentication, such as password reset.
Customization
Laravel’s authentication system is highly customizable and allows you to tailor IT to your application’s needs. You can customize various aspects, such as the authentication guards, authentication providers, password reset, and more.
Authentication Guards
Laravel provides multiple authentication guards out of the box, including web
and api
. The web
guard is typically used for authenticating users who access your web application via a web browser. The api
guard, on the other hand, is used for authenticating requests made to your application’s API endpoints.
You can customize the authentication guards by modifying the guards
configuration file, which can be found in the config/auth.php
directory. In this file, you can define additional guards or modify the existing ones according to your requirements.
Authentication Providers
Laravel’s authentication system uses providers to retrieve user information from the database or other sources. By default, Laravel uses the EloquentUserProvider
to retrieve user information from the database. However, you can create custom authentication providers to retrieve user information from alternative sources, such as external APIs or LDAP servers.
You can customize the authentication providers by modifying the providers
configuration file, which can also be found in the config/auth.php
directory. In this file, you can define additional providers or modify the existing ones as per your application’s needs.
FAQs
Q: Can I use a different database for authentication?
A: Yes, you can configure Laravel to use a different database for authentication by modifying the database
configuration file (config/database.php
). In this file, you can specify separate connections or modify the existing connection for authentication.
Q: How can I add additional fields to the registration form?
A: To add additional fields to the registration form, you need to modify the view and controller responsible for registration. Open the resources/views/auth/register.blade.php
file to add HTML markup for the new fields. Additionally, modify the RegisterController
to handle the additional fields during form submission and user creation.
Q: How can I customize the password reset functionality?
A: Laravel provides a convenient way to customize the password reset functionality. You can modify the views and controllers responsible for password reset, which can be found in the resources/views/auth/passwords
directory and the app/Http/Controllers/Auth/
directory, respectively. You can add additional validation rules, customize the email template, or even change the password reset logic as per your requirements.