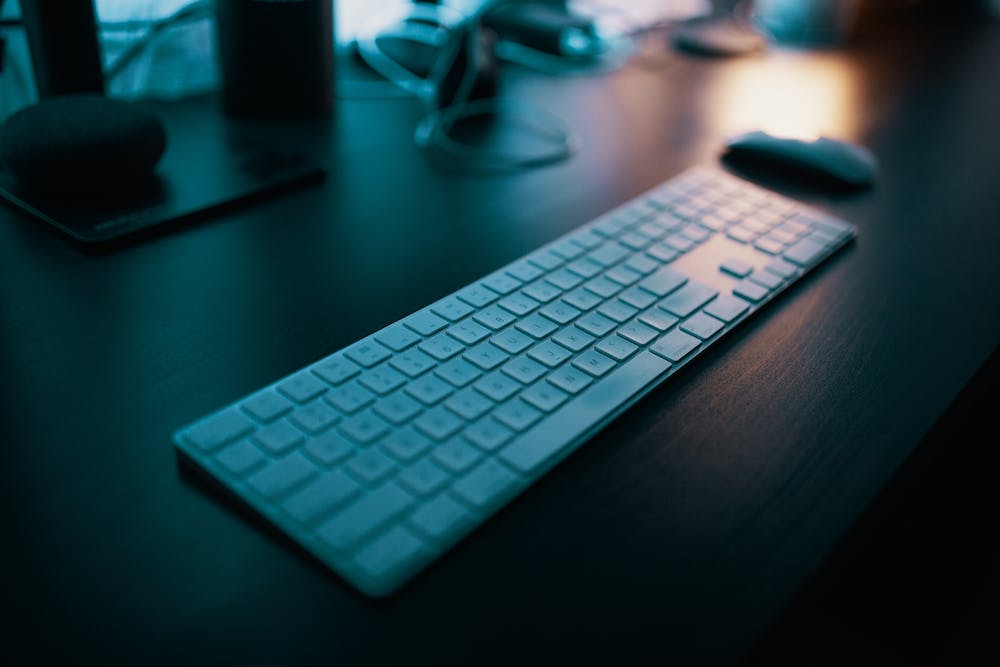
JavaScript is a powerful programming language that provides a multitude of features and functions for developers to utilize in their projects. One such function is the ‘wait()’ function, which allows you to pause the execution of your code for a specified period of time. In this guide, we will take an in-depth look at how to use the ‘wait()’ function in JavaScript, along with some common use cases and potential FAQs.
Using the wait() Function
The ‘wait()’ function is not a built-in function in JavaScript, but IT can be easily implemented using the ‘setTimeout()’ function. The ‘setTimeout()’ function is used to execute a piece of code after a specified timeout period.
function wait(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
In the above code snippet, we create a function named ‘wait()’ that takes a parameter ‘ms’ representing the number of milliseconds to wait. The function returns a Promise that will resolve after the specified timeout period using the ‘setTimeout()’ function.
Now, let’s look at an example of how to use the ‘wait()’ function in practice:
async function myFunction() {
console.log("Hello");
await wait(2000);
console.log("World!");
}
In this example, the code will log “Hello” to the console and then wait for 2000 milliseconds (or 2 seconds) before logging “World!”. This allows us to introduce a delay in the execution of our code, which can be useful in various scenarios.
Common Use Cases
The ‘wait()’ function can be particularly useful in scenarios such as:
- Creating a delay between sequential actions in a script.
- Animating elements on a webpage with timed transitions.
- Simulating asynchronous behavior when testing code.
By introducing delays with the ‘wait()’ function, you can control the timing and synchronization of different parts of your code.
FAQs
Q: Can I use the ‘wait()’ function with non-numeric arguments?
A: No, the ‘wait()’ function expects a numeric argument representing the number of milliseconds to wait. Passing non-numeric arguments will result in unexpected behavior or errors.
Q: Can I cancel a wait() operation?
A: The ‘wait()’ function implemented using ‘setTimeout()’ cannot be directly canceled. However, you can store the timeout identifier returned by ‘setTimeout()’ and use ‘clearTimeout()’ to cancel the waiting operation.
Q: Are there any alternatives to the wait() function?
A: Yes, there are alternative libraries and functions available, such as the ‘setInterval()’ function, which repeatedly executes a function with a fixed time delay between each call. Additionally, libraries like ‘async’ and ‘await’ can provide more complex control flows for asynchronous operations.
Q: Are there any downsides to using the wait() function?
A: While the ‘wait()’ function is useful in certain scenarios, excessive use of delays can lead to poor user experiences, especially in web applications. Always consider the context and purpose of introducing delays in your code.
Q: Is the wait() function compatible with all browsers?
A: The ‘wait()’ function itself is not a standardized JavaScript function, but since IT is implemented using the ‘setTimeout()’ function, which is widely supported by all major browsers, IT should work in most modern environments.