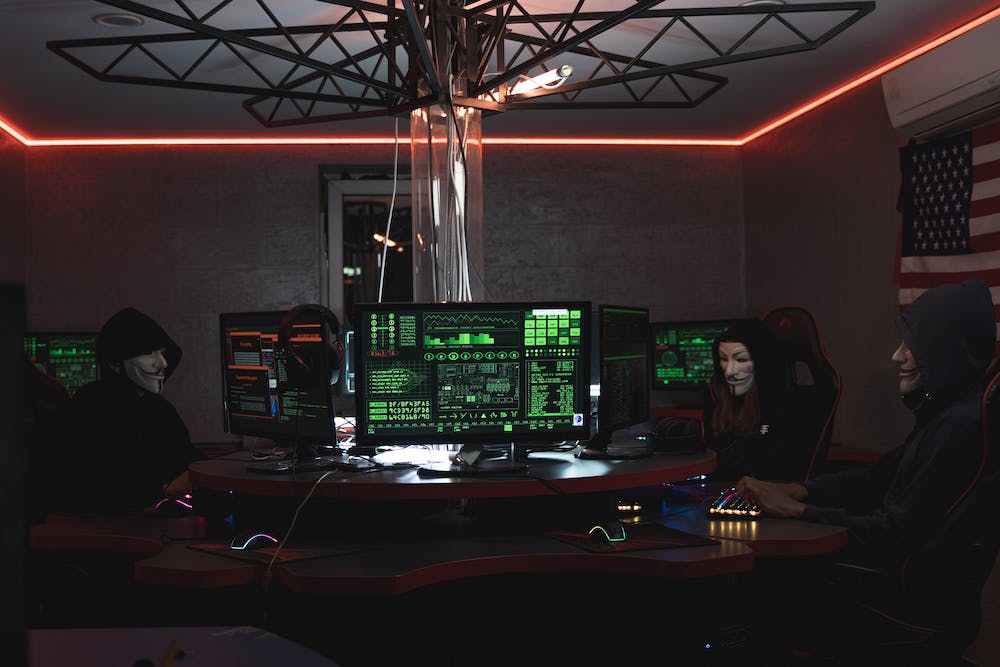
PHP session management is an essential aspect of web development, allowing developers to maintain user data across multiple page requests. IT enables websites to remember information about users, such as login credentials or shopping cart contents, during their browsing sessions. In this beginner’s guide, we will explore the basics of PHP session management, outlining its importance, usage, and common practices.
Why is PHP Session Management Important?
Web sessions play a crucial role in enhancing user experience by providing personalized and customized services. PHP session management allows developers to create a unique session ID for each user, which can be used to store and retrieve their data as they navigate through different pages on a Website.
Starting a Session
In PHP, you can start a session using the session_start()
function. IT must be called before any output is sent to the browser. Typically, the session start function is placed at the top of the PHP script or in a separate include file.
<?php
session_start();
?>
Storing and Accessing Session Data
To store data in a session, you can utilize the $_SESSION
superglobal array. IT allows you to create named variables that can be accessed across different pages during the same session. For example, to store a user’s name, you would do the following:
<?php
$_SESSION['name'] = 'John';
?>
To access the stored value on another page, you would utilize the same session variable:
<?php
echo $_SESSION['name']; // Outputs 'John'
?>
Ending a Session
Ending a session is important to free up system resources and ensure proper session management. You can use the session_destroy()
function to destroy the current session and unset all session variables:
<?php
session_destroy();
?>
Checking if a Session Variable Exists
You can verify if a session variable exists by using the isset()
function. IT returns true
if the variable exists and false
otherwise. Here’s an example:
<?php
if (isset($_SESSION['name'])) {
echo 'Session variable exists!';
} else {
echo 'Session variable does not exist!';
}
?>
Session Timeouts
Sessions have a timeout period, which determines how long a user’s session data will be stored on the server after inactivity. By default, the session variables are kept for 24 minutes after the last request. You can modify this value in the PHP configuration file (php.ini) by adjusting the session.gc_maxlifetime
option.
Common Session Security Practices
Secure session management is vital to protect user data and prevent unauthorized access. Here are some best practices:
- Always start sessions on secure pages (HTTPS) to prevent eavesdropping on the session data.
- Regenerate the session ID periodically or after significant events to prevent session fixation attacks.
- Avoid storing sensitive information directly in session variables; instead, use encryption or hashing techniques to secure the data.
- Prevent session hijacking by enabling session.cookie_httponly in the PHP configuration file, which restricts cookie access to HTTP requests.
- Set appropriate session cookie parameters like
session.cookie_secure = 1
for ensuring cookies are only sent over secure connections.
Frequently Asked Questions (FAQs):
Q: Can session data be accessed by users?
A: No, session data is stored on the server and is not accessible by users directly. Only the session ID, typically stored in a cookie or passed through GET or POST requests, is sent to the user’s browser.
Q: Can I store arrays or objects in session variables?
A: Yes, PHP allows you to store arrays and objects directly into session variables. However, serialization and deserialization may be required to maintain the data’s integrity.
Q: What happens when a user’s session expires?
A: When a user’s session expires due to inactivity or being manually destroyed, the session data is deleted from the server. The user will need to start a new session, and previously stored session data will no longer be accessible.
Q: Can session data be stored in a database instead of server memory?
A: Yes, PHP provides options to store session data in a database instead of server memory. This allows for scalability and sharing sessions across multiple servers in a clustered environment. You can configure PHP to handle session storage using database backends.
In conclusion, PHP session management is a fundamental tool for maintaining user-specific data throughout a browsing session. By understanding its usage and implementing proper security practices, developers can create dynamic and personalized web applications that enhance user experience.