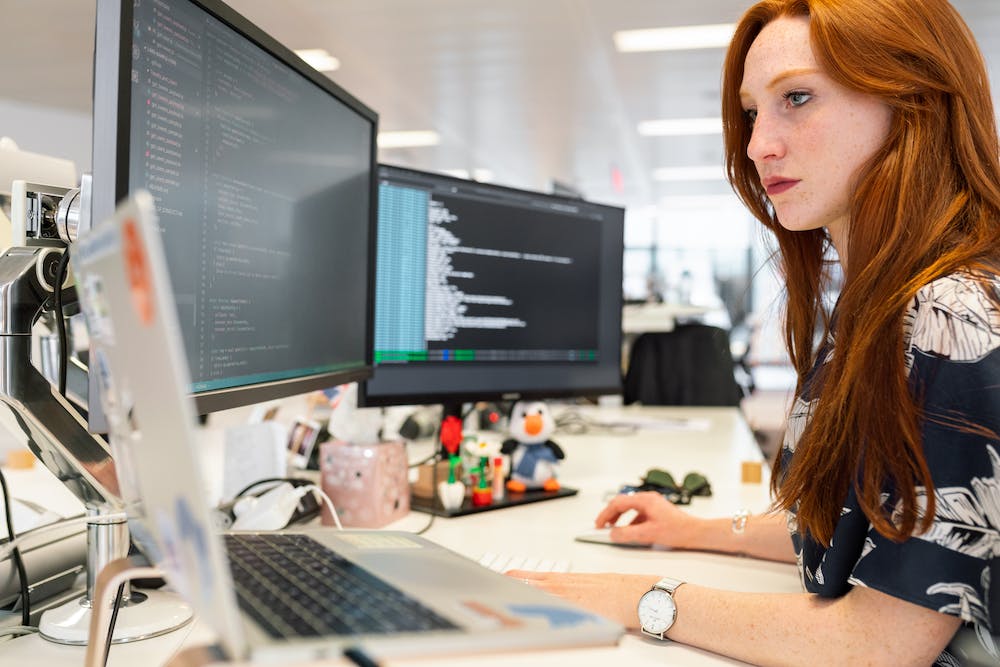
PHP is a widely used programming language for web development. IT is known for its simplicity and flexibility, making IT a popular choice for beginners. One of the key functions in PHP is the count() function. In this guide, we will explore the basics of the PHP count() function and how IT can be used in various scenarios.
The count() function in PHP is used to count the number of elements in an array or the properties in an object. IT returns the number of elements in an array or the number of properties in an object. This function is extremely useful when you need to know the size of an array or the number of properties in an object dynamically.
To use the count() function, you simply pass the array or object as a parameter to the function. Here’s a basic example:
“`php
$fruits = array(“Apple”, “Banana”, “Orange”);
$count = count($fruits);
echo “The number of fruits is: ” . $count;
?>
“`
This will output:
“`
The number of fruits is: 3
“`
As you can see, the count() function returned the number of elements in the $fruits array, which is 3 in this case.
The count() function can also be used to count the number of properties in an object. Let’s take a look at an example:
“`php
class Person {
public $name;
public $age;
}
$person = new Person();
$person->name = “John”;
$person->age = 25;
$count = count((array)$person);
echo “The number of properties in the person object is: ” . $count;
?>
“`
This will output:
“`
The number of properties in the person object is: 2
“`
In this example, we created a Person class with two properties: name and age. We then created an instance of the Person class and assigned values to its properties. We converted the object to an array using the (array) type casting, and then passed IT to the count() function. The count() function returned the number of properties in the object, which is 2 in this case.
The count() function can also be used in more complex scenarios. For example, you can use IT to count the number of elements that satisfy a certain condition in an array. Let’s see an example:
“`php
$numbers = array(2, 4, 6, 8, 10);
$count = count(array_filter($numbers, function($number) {
return $number % 2 == 0;
}));
echo “The number of even numbers is: ” . $count;
?>
“`
This will output:
“`
The number of even numbers is: 5
“`
In this example, we used the array_filter() function to filter the elements of the $numbers array that satisfy the condition of being even numbers. We then passed the filtered array to the count() function, which returned the number of elements in the filtered array, which is 5 in this case.
FAQs
Q: Can the count() function be used with multidimensional arrays?
A: Yes, the count() function can be used with multidimensional arrays. IT will return the number of elements in the top-level array.
Q: Can the count() function be used to count the number of elements in a string?
A: No, the count() function cannot be used to count the number of elements in a string. IT is specifically designed for arrays and objects.
Q: Are there any performance considerations when using the count() function?
A: The count() function is generally efficient and does not have significant performance considerations. However, if you are working with large arrays or objects, calling the count() function repeatedly may have an impact on performance. In such cases, IT is recommended to store the count result in a variable instead of calling the function multiple times.
In conclusion, the count() function is a powerful tool in PHP for counting the elements in an array or the properties in an object. IT is easy to use and provides valuable information for dynamic programming. Whether you are a beginner or an experienced developer, understanding the count() function will undoubtedly help you in your PHP endeavors.