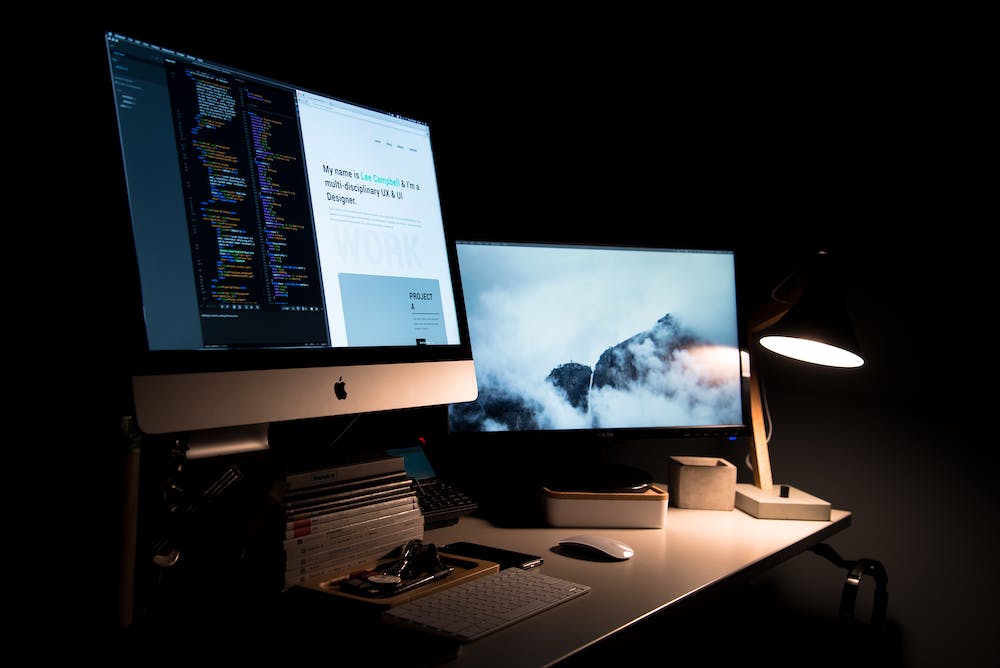
XML (eXtensible Markup Language) is a widely used language for structuring and storing data. IT provides a way to describe the structure of data in a format that is both human-readable and machine-readable. As a beginner, understanding how to parse XML can be challenging but with the help of libraries like xml2js, IT becomes much easier. In this article, we will explore a beginner’s guide to parsing XML with xml2js and provide answers to some frequently asked questions.
What is xml2js?
Xml2js is a JavaScript library that allows you to convert XML into JavaScript objects. IT provides a simple and intuitive API for parsing and manipulating XML data. Whether you want to extract specific values from an XML document or convert XML data into a different format, xml2js can be your go-to library.
Installing xml2js
Before we can start using xml2js, we need to install IT. You can easily install IT using npm, the package manager for Node.js. Open your terminal or command prompt and run the following command:
$ npm install xml2js
Basic XML Parsing with xml2js
Once xml2js is installed, we can begin parsing XML data. Let’s start with a simple example:
const xml2js = require('xml2js');
const xmlData = `
<person>
<name>John Doe</name>
<age>30</age>
</person>
`;
xml2js.parseString(xmlData, (err, result) => {
if (err) {
console.error(err);
} else {
console.log(result);
}
});
In the above code, we import the xml2js library and define some XML data as a string. We then use the parseString
function provided by xml2js to convert the XML data into a JavaScript object. The resulting object is logged to the console.
Accessing Parsed XML Data
Once we have parsed the XML data, accessing specific values becomes straightforward. xml2js converts the XML tags and their values into properties of the resulting JavaScript object. For example, let’s say we have the following XML data:
const xmlData = `
<person>
<name>John Doe</name>
<age>30</age>
</person>
`;
xml2js.parseString(xmlData, (err, result) => {
const name = result.person.name[0];
const age = result.person.age[0];
console.log('Name:', name);
console.log('Age:', age);
});
In this code snippet, we access the values of the name
and age
tags by simply referencing them as properties of the result
object. Since xml2js converts the values to arrays, we use index 0 to access the first (and only) item.
Handling Attributes in XML
XML tags can have attributes along with their values. To access these attributes, we use a slightly different syntax. Consider the following XML data:
const xmlData = `
<person id="1">
<name>John Doe</name>
<age>30</age>
</person>
`;
xml2js.parseString(xmlData, (err, result) => {
const id = result.person.$attributes.id;
console.log('ID:', id);
});
In this code snippet, we access the id
attribute of the person
tag by referencing result.person.$attributes.id
. The $attributes
property contains all the attributes of the given tag.
FAQs
Q: Can xml2js parse XML files from a URL?
A: Yes, xml2js can parse XML files from a URL. You can use libraries like axios
or fetch
to fetch the XML data from the URL and then pass IT to xml2js for parsing.
Q: How can I handle errors while parsing XML with xml2js?
A: When calling the parseString
function, you can use the callback function to handle errors. If an error occurs during parsing, the err
parameter will contain the error object.
Q: Can xml2js be used in a browser environment?
A: No, xml2js is primarily designed for use in Node.js applications. For parsing XML in a browser environment, you can use alternative libraries like DOMParser
or fast-xml-parser
.
Q: Can xml2js handle large XML files?
A: Yes, xml2js can handle large XML files. IT uses a streaming parser that doesn’t load the entire XML document into memory, making IT memory-efficient for processing large XML files.
Q: Does xml2js support XML namespaces?
A: Yes, xml2js has built-in support for XML namespaces. When accessing tag values, you need to include the namespace prefix along with the tag name.
With xml2js, parsing XML becomes accessible and manageable even for beginners. Its intuitive API and support for handling various XML structures make IT a reliable choice for XML parsing tasks. As you gain more experience, you can explore additional features provided by xml2js to further enhance your XML parsing workflow.