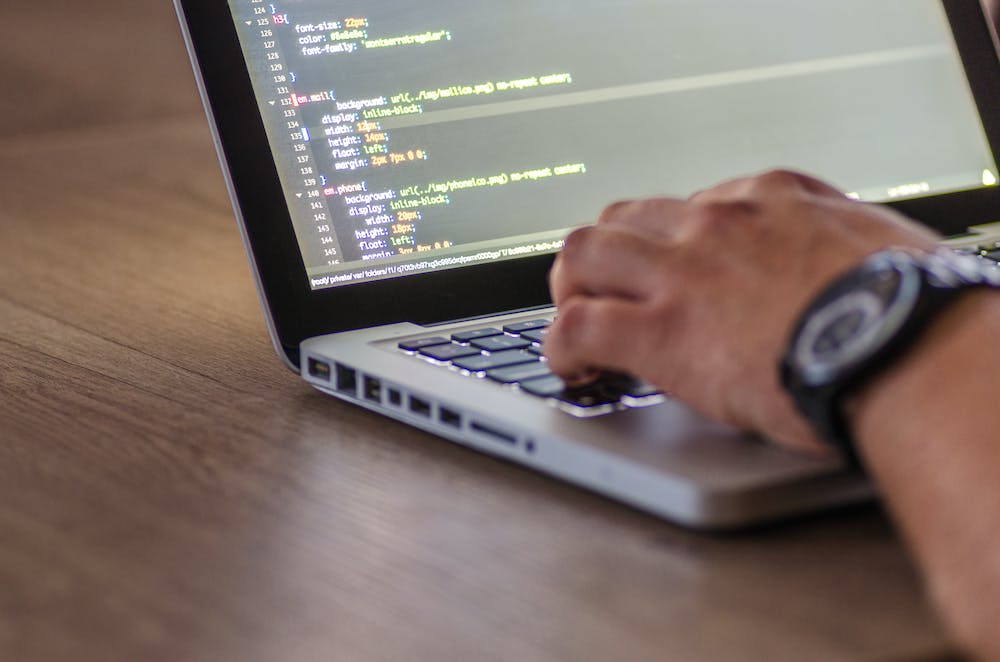
MySQLi is a powerful extension of the PHP programming language that allows developers to easily interact with MySQL databases. One of the most fundamental operations in database programming is retrieving data from a database table. In this guide, we will explore the mysqli_fetch_row function, which is commonly used to retrieve data from a MySQL database using PHP.
The mysqli_fetch_row function is used to fetch a single row from the result set returned by a SELECT statement. IT returns an indexed array that represents the fetched row, with each element in the array corresponding to the value in each column of the row.
To use the mysqli_fetch_row function, you first need to establish a connection to your MySQL database using the mysqli_connect function. Once you have a connection, you can execute a SELECT statement using the mysqli_query function and retrieve the result set.
Here’s a basic example that demonstrates how to use mysqli_fetch_row to retrieve data from a MySQL database:
$connection = mysqli_connect("localhost", "username", "password", "database_name");
$query = "SELECT * FROM users";
$result = mysqli_query($connection, $query);
while ($row = mysqli_fetch_row($result)) {
// process each row of data
echo "User ID: " . $row[0] . " | Username: " . $row[1] . " | Email: " . $row[2] . "<br>";
}
mysqli_close($connection);
In the above example, we assume a table named “users” with three columns: “id”, “username”, and “email”. The mysqli_fetch_row function is used within a while loop to iterate over each row in the result set, fetching the row as an indexed array and processing IT as needed.
Now let’s answer some frequently asked questions about mysqli_fetch_row:
FAQs
Q: Can I use mysqli_fetch_row to fetch multiple rows at once?
A: No, mysqli_fetch_row retrieves one row at a time. You need to call the function repeatedly within a loop to fetch multiple rows.
Q: What happens if there are no more rows to fetch?
A: When there are no more rows to fetch, mysqli_fetch_row returns NULL.
Q: How can I access the values in each column of a fetched row?
A: The values can be accessed using numeric indices, starting from 0. For example, $row[0] refers to the first column in the row, $row[1] refers to the second column, and so on.
Q: Is mysqli_fetch_row case-sensitive?
A: Yes, mysqli_fetch_row is case-sensitive. Make sure to match the column names exactly as they appear in your database table.
Q: Can I use mysqli_fetch_row with prepared statements?
A: Yes, you can use mysqli_fetch_row with prepared statements. However, IT‘s more common to use other functions like mysqli_fetch_assoc or mysqli_fetch_array when working with prepared statements.
By using the mysqli_fetch_row function, you can easily retrieve data from a MySQL database using PHP. Remember to establish a connection, execute a SELECT statement, and iterate over the result set to fetch and process the data. With this knowledge, you can start building dynamic web applications that interact with a MySQL database.