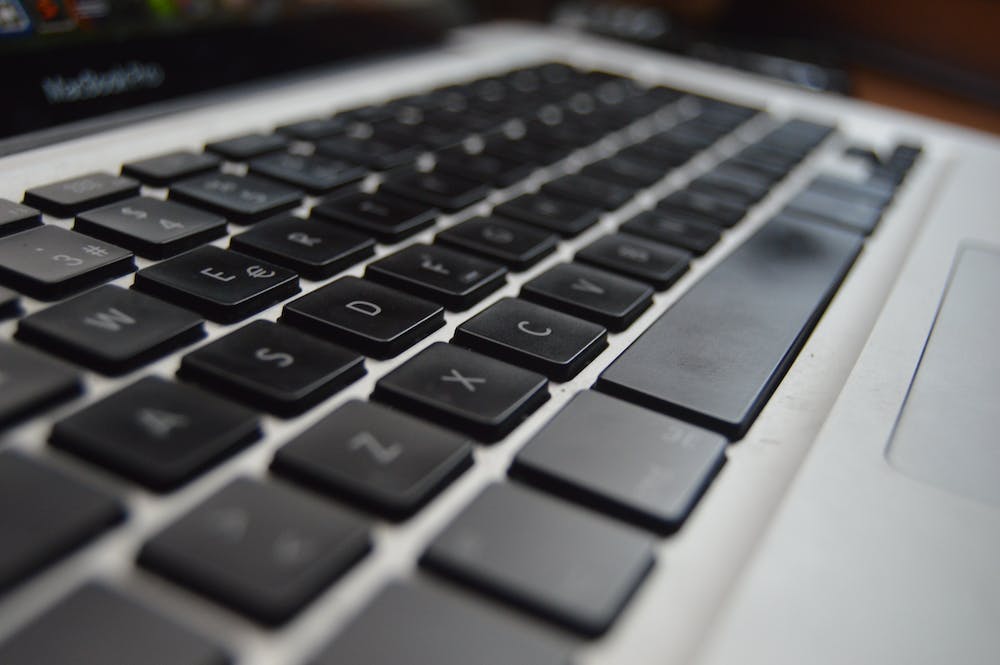
Laravel is a popular PHP framework that is widely used for web development. IT provides developers with an elegant and intuitive way to build and maintain web applications. If you are new to Laravel or PHP frameworks in general, this guide will help you get started with Laravel and learn the basics of this powerful tool.
Installation
To begin using Laravel, you need to have PHP installed on your system. Laravel requires PHP version 7.2.5 or higher. You can check your PHP version by running the following command in your terminal:
php -v
If you don’t have PHP installed or need to update IT, you can download IT from the official PHP Website. Once you have PHP installed, you can install Laravel using Composer, which is a dependency management tool for PHP. Run the following command in your terminal to install Laravel:
composer global require laravel/installer
This will install the Laravel installer globally on your system, allowing you to create new Laravel projects from anywhere. Once the installation is complete, you can create a new Laravel project by running the following command:
laravel new project-name
Replace “project-name” with the desired name for your project. This command will create a new Laravel project in a directory with the specified name.
Development Environment
Laravel has a built-in development server that you can use while developing your application. To start the development server, navigate to the root directory of your Laravel project in the terminal and run the following command:
php artisan serve
This will start the development server, and you can access your Laravel application by visiting http://localhost:8000 in your web browser. Any changes you make to your application will be reflected immediately, and you can see the results in your browser.
By default, Laravel uses the SQLite database for local development. However, you can configure Laravel to use other databases such as MySQL or PostgreSQL. Laravel provides a clean and simple API for interacting with databases, making IT easy to perform database operations in your application.
Routing
Laravel uses a routing system to map incoming requests to the appropriate controller method. You can define routes using a simple and expressive syntax. Routes are typically defined in the “routes/web.php” file for web applications.
Here is an example of a basic route definition:
Route::get('/welcome', function () { return 'Welcome to Laravel!'; });
This route responds to GET requests to the “/welcome” URL and returns the specified string. You can define routes for different HTTP methods such as GET, POST, PUT, DELETE, etc., and specify the corresponding controller method to handle the request.
Views and Blade Templates
Laravel uses a template engine called Blade, which provides a convenient way to organize and display your application’s HTML. Blade templates are stored in the “resources/views” directory and have a “.blade.php” file extension.
Here is an example of a simple Blade template:
<html>
<head>
<title>{{ $title }}</title>
</head>
<body>
<h1>{{ $message }}</h1>
</body>
</html>
In this example, the “title” and “message” variables are passed to the template and displayed using double curly braces. Blade templates allow you to easily include other templates, create reusable components, and use control structures such as loops and conditionals.
FAQs:
Q: What are the advantages of using Laravel?
A: Laravel provides a wide range of features and tools that make web development easier and more efficient. Some of the advantages of using Laravel include its expressive syntax, powerful ORM (Object-Relational Mapping), built-in authentication and authorization system, caching mechanisms, and robust routing system.
Q: Is Laravel suitable for beginners?
A: Yes, Laravel is suitable for beginners as IT has a gentle learning curve and provides comprehensive documentation. Its intuitive syntax and built-in features make IT easy for beginners to get started with web development using Laravel.
Q: Can I use Laravel with other front-end frameworks?
A: Yes, you can use Laravel with other front-end frameworks such as Vue.js or React. Laravel provides a preset for Vue.js, which allows you to quickly scaffold a project with Laravel and Vue.js integration. You can also use Laravel as a backend API for your JavaScript-based front-end applications.
Q: Is Laravel suitable for enterprise-level applications?
A: Yes, Laravel is widely used for developing enterprise-level applications. Its robust architecture, extensive feature set, and scalability make IT an excellent choice for building large-scale web applications.
Q: Is Laravel free to use?
A: Yes, Laravel is an open-source framework released under the MIT license, which means IT is free to use, modify, and distribute.
In conclusion, Laravel is a powerful PHP framework that provides developers with a flexible and efficient way to build web applications. With its expressive syntax, elegant template engine, and rich set of features, Laravel is a great choice for both beginners and experienced developers. By following this beginner’s guide, you can get started with Laravel and begin your journey into modern web development.