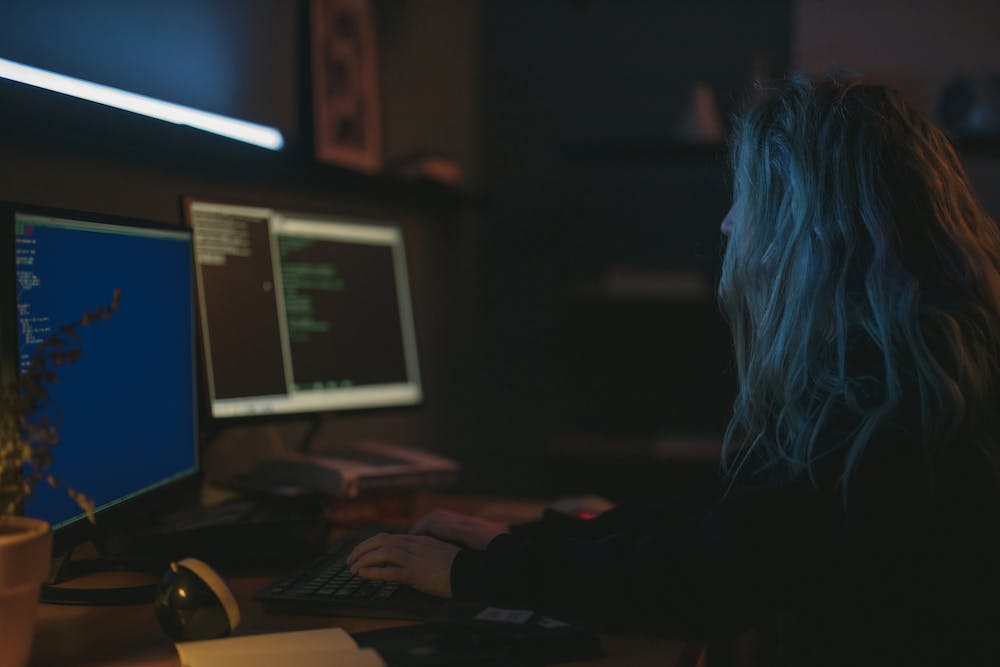
JavaScript is a dynamic programming language that is widely used for enhancing the interactivity and functionality of websites. As a beginner, learning JavaScript can be both exciting and challenging. In this guide, we will take a closer look at the basics of JavaScript, including its syntax, variables, data types, and functions. By the end of this article, you will have a solid foundation to start creating your own interactive web applications!
Syntax
Before diving into the various concepts of JavaScript, let’s understand the basic syntax of the language. In JavaScript, statements are typically ended with a semicolon. Comments can be added using the ‘//’ (for single-line comments) or ‘/* */’ (for multi-line comments) syntax. JavaScript is case-sensitive, meaning that ‘myVariable’ and ‘myvariable’ are considered as two different variables.
Variables
In JavaScript, you can declare variables using the ‘var’, ‘let’, or ‘const’ keyword, followed by the variable name. For example:
var myVariable = 10;
Here, we have declared a variable named ‘myVariable’ and assigned IT the value of 10. The ‘var’ keyword is used to declare a variable that can be accessed throughout the function scope. On the other hand, ‘let’ and ‘const’ are used to declare block-scoped variables. The difference between ‘let’ and ‘const’ is that the value of a ‘let’ variable can be reassigned, whereas the value of a ‘const’ variable remains constant.
Data Types
JavaScript has several built-in data types, including:
- Numbers: used to represent numeric values (e.g., 10, 3.14).
- Strings: used to represent textual data (e.g., “Hello, World!”).
- Booleans: used to represent true/false values.
- Arrays: used to store multiple values in a single variable.
- Objects: used to store key-value pairs.
- Null: used to represent the absence of a value.
- Undefined: used to indicate that a variable has been declared but has no value assigned to IT.
Functions
Functions are an essential building block in JavaScript. They allow you to define reusable blocks of code that can be executed whenever needed. A function can have parameters (placeholders for values) and can return a value. Here’s an example:
function greet(name) {
console.log("Hello, " + name + "!");
}
greet("John"); // Output: Hello, John!
In this example, we have defined a function named ‘greet’ that takes a parameter called ‘name’. IT then logs a greeting message to the console using the provided name. When we call the function with the argument “John”, IT prints “Hello, John!” to the console.
FAQs
Q: Can JavaScript be used on the server-side?
A: Yes, JavaScript can be used on the server-side as well. Node.js is a popular runtime environment that allows you to run JavaScript code on a server, enabling you to build powerful server-side applications.
Q: What is the difference between ‘==’ and ‘===’ in JavaScript?
A: ‘==’ is used for loose equality, meaning that IT checks for equality of values without considering the data types. ‘===’ is used for strict equality, meaning that IT checks for equality of values and data types.
Q: Is JavaScript the same as Java?
A: No, JavaScript and Java are two different programming languages. Despite the similar name, they have different syntax, use cases, and application areas.
Q: Can I use JavaScript to manipulate HTML elements?
A: Absolutely! JavaScript provides powerful DOM (Document Object Model) manipulation capabilities that allow you to dynamically change the content, style, and behavior of HTML elements on a webpage.
With this beginner’s guide, you should now have a good understanding of the basics of JavaScript. Remember, practice is key to mastering any programming language. Keep experimenting, building small projects, and exploring more advanced concepts to become a proficient JavaScript developer!