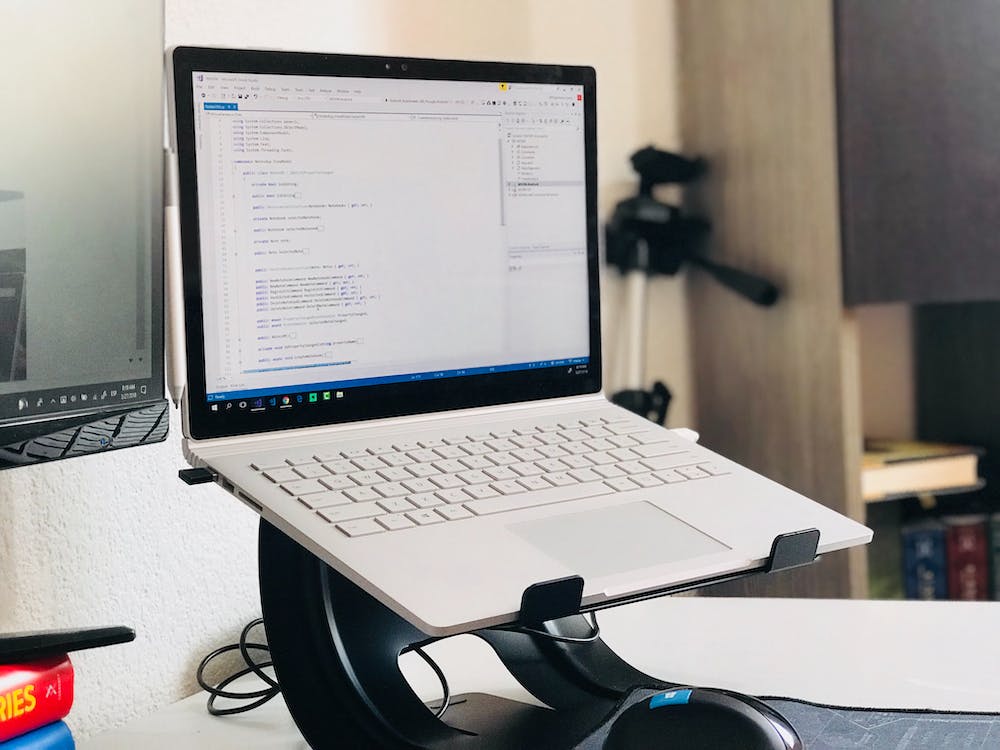
A Beginner’s Guide to JavaScript Promises
Introduction
JavaScript is a versatile programming language that allows developers to create interactive websites and web applications. IT is commonly used for tasks such as form validation, DOM manipulation, and handling asynchronous operations. Asynchronous operations, such as requesting data from an API or reading a file, can be tricky to handle in JavaScript, as they can cause blocking issues. JavaScript promises provide a solution to this problem by allowing developers to write code that can handle asynchronous tasks efficiently.
What are JavaScript Promises?
In simple terms, a promise in JavaScript represents the eventual completion or failure of an asynchronous operation and its resulting value. IT is an object that may produce a single value in the future, either resolved or rejected. The promise state can be one of three states: pending, fulfilled, or rejected.
Using Promises
To use promises in JavaScript, we first need to create a promise object. We can do this using the Promise constructor, which takes a function as its argument. This function is called the executor function and contains the asynchronous operation we want to perform.
The executor function has two parameters: resolve and reject. These parameters are also functions that can be called to fulfill or reject the promise. We can use them to indicate the successful completion or failure of our asynchronous operation.
const promise = new Promise((resolve, reject) => {
// Asynchronous operation
// If successful, call resolve with the result
// If failed, call reject with an error
});
Once we have created a promise object, we can use methods like `.then()` and `.catch()` to handle the fulfillment or rejection of the promise. The `.then()` method takes a callback function that will be executed when the promise is resolved, and the `.catch()` method takes a callback function that will be executed when the promise is rejected.
promise
.then((result) => {
// Code to handle the successful fulfillment of the promise
})
.catch((error) => {
// Code to handle the rejection of the promise
});
Chaining Promises
One of the most powerful features of promises is the ability to chain them together. This allows us to perform multiple asynchronous operations in a specific order and handle the results sequentially.
We can chain promises using the `.then()` method. The callback function passed to the `.then()` method can return a new promise, which will be resolved before moving to the next `.then()` in the chain. This helps to create a more readable and organized code structure.
promise
.then((result) => {
// Code to handle the successful fulfillment of the first promise
return new Promise((resolve, reject) => {
// Asynchronous operation
// If successful, call resolve with the result
// If failed, call reject with an error
});
})
.then((result) => {
// Code to handle the successful fulfillment of the second promise
return new Promise((resolve, reject) => {
// Asynchronous operation
// If successful, call resolve with the result
// If failed, call reject with an error
});
})
.catch((error) => {
// Code to handle any errors in the promise chain
});
Conclusion
JavaScript promises are a powerful tool for handling asynchronous operations in a more organized and efficient way. They allow developers to write code that can handle the fulfillment or rejection of promises, making IT easier to manage complex asynchronous tasks.
FAQs
Q: Can promises be used in all modern browsers?
A: Yes, promises are supported in all modern browsers, including Chrome, Firefox, Safari, and Edge. However, for older browsers that do not support promises natively, there are polyfills available that provide the same functionality.
Q: Can I convert callback-based functions to promises?
A: Yes, you can convert callback-based functions to promises using the `util.promisify()` function in Node.js or by manually wrapping the function with a promise. This can make your code more reliable and easier to read and maintain.
Q: Are promises better than callbacks for handling asynchronous operations?
A: Promises provide a more structured and readable way to handle asynchronous operations compared to traditional callback functions. They allow you to chain multiple asynchronous tasks together and handle errors more effectively. However, the choice between promises and callbacks depends on the specific use case and personal preference.
In conclusion, JavaScript promises are a valuable addition to any developer’s toolkit when working with asynchronous tasks. Understanding how to create and effectively use promises can greatly enhance the quality and performance of your JavaScript code.