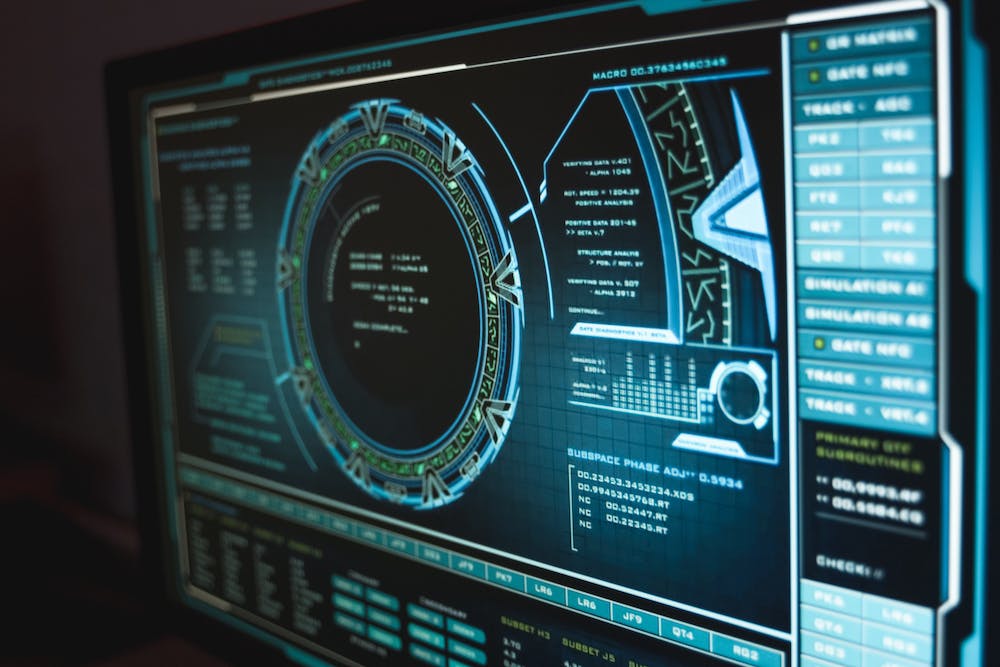
In the world of web development, JavaScript is a powerful programming language that can be used to create dynamic and interactive web applications. One popular use case for JavaScript is creating a to-do list application. In this beginner’s guide, we will walk you through the process of creating a simple to-do list using JavaScript.
Getting Started
Before we dive into the code, let’s first understand the basic structure of a to-do list application. A to-do list typically consists of a list of tasks that need to be completed, along with the ability to add new tasks, mark tasks as completed, and delete tasks.
To create a to-do list with JavaScript, we will need to use HTML for the structure of the list and the input field for adding new tasks, and JavaScript to handle the logic of adding, marking, and deleting tasks.
Creating the HTML Structure
Let’s start by creating the HTML structure for our to-do list. We will use an unordered list (<ul>
) to store the tasks, and an input field (<input>
) along with a button to add new tasks.
<h2>To-Do List</h2>
<input type="text" id="taskInput">
<button onclick="addTask()">Add</button>
<ul id="taskList"></ul>
Adding JavaScript Logic
Now that we have the basic HTML structure in place, let’s add the JavaScript logic to handle adding tasks to the list. We will also add the ability to mark tasks as completed and delete tasks.
<script>
function addTask() {
var taskInput = document.getElementById('taskInput');
var taskList = document.getElementById('taskList');
var task = taskInput.value;
if (task !== '') {
var li = document.createElement('li');
li.appendChild(document.createTextNode(task));
taskList.appendChild(li);
taskInput.value = '';
li.onclick = function() {
if (this.style.textDecoration === 'line-through') {
this.style.textDecoration = 'none';
} else {
this.style.textDecoration = 'line-through';
}
};
li.ondblclick = function() {
this.remove();
};
}
}
</script>
With the above JavaScript code, we have created a function addTask()
that retrieves the task input, creates a new list item (<li>
), appends the task text, and appends IT to the task list. We have also added the ability to mark tasks as completed by clicking on them, and the ability to delete tasks by double-clicking on them.
Conclusion
Congratulations! You have now successfully created a simple to-do list application using JavaScript. This is just a starting point, and you can continue to build upon this foundation by adding more features such as filtering tasks, saving tasks to local storage, or even integrating with a backend server. The possibilities are endless with JavaScript!
FAQs
Q: Can I style my to-do list using CSS?
A: Absolutely! You can use CSS to style your to-do list with custom fonts, colors, and layout to make it visually appealing and user-friendly.
Q: How can I save my to-do list tasks for later use?
A: You can use browser local storage or a backend database to save your to-do list tasks for later use. This would require additional JavaScript and possibly server-side programming.
Q: Are there any libraries or frameworks that can help with building to-do list applications?
A: Yes, there are many JavaScript libraries and frameworks such as React, Vue, or Angular that can help streamline the process of building to-do list applications and provide additional features and functionality.