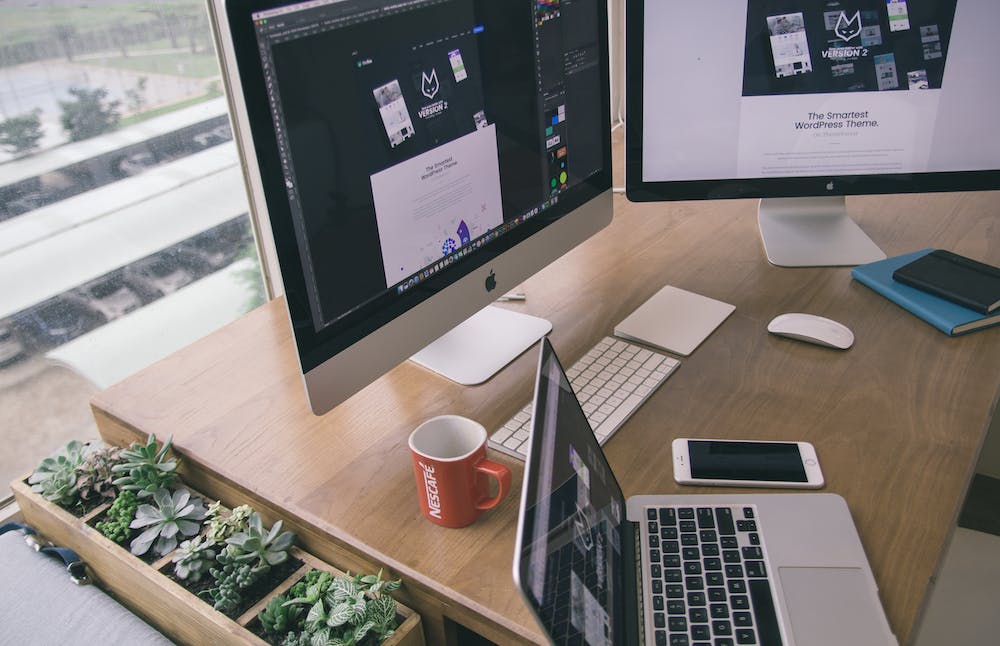
PHP (Hypertext Preprocessor) is a widely used server-side scripting language. IT is especially popular for web development due to its ease of use, flexibility, and extensive functionality. In this article, we will explore seven jaw-dropping PHP programs that will revolutionize your coding skills.
1. Fibonacci Sequence Generator
The Fibonacci sequence is a fascinating mathematical pattern where each number is the sum of the two preceding ones (starting from 0 and 1). writing a PHP program to generate the Fibonacci sequence is a great exercise for understanding loops and recursion.
Example:
<?php
// Function to generate Fibonacci sequence
function fibonacci($n) {
$sequence = array(0, 1);
for ($i = 2; $i < $n; $i++) {
$sequence[$i] = $sequence[$i-1] + $sequence[$i-2];
}
return $sequence;
}
// Generate Fibonacci sequence with 10 numbers
$fibonacciSequence = fibonacci(10);
// Print the sequence
foreach ($fibonacciSequence as $number) {
echo $number . " ";
}
?>
This program generates the Fibonacci sequence up to a given number (in this case, 10) and outputs IT to the browser.
2. Prime Number Checker
A prime number is a positive integer greater than 1 that has no divisors other than 1 and itself. Building a PHP program to check whether a number is prime or not is an excellent challenge for strengthening your logical thinking.
Example:
<?php
// Function to check if a number is prime
function isPrime($number) {
if ($number < 2) {
return false;
}
for ($i = 2; $i <= sqrt($number); $i++) {
if ($number % $i == 0) {
return false;
}
}
return true;
}
// Check if the number 17 is prime
$number = 17;
if (isPrime($number)) {
echo $number . " is a prime number.";
} else {
echo $number . " is not a prime number.";
}
?>
This program checks if a given number (in this case, 17) is a prime number and prints the result to the browser.
3. Word Count Analyzer
Counting the occurrences of words in a text is a common task in various applications. A PHP program that analyzes the word count of a text can be extremely useful, especially for text processing and natural language processing tasks.
Example:
<?php
// Text to analyze
$text = "Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed eu ante rutrum, facilisis metus vel, dapibus nibh. Ut sed vulputate dui.";
// Function to analyze word count
function wordCount($text) {
$words = str_word_count($text, 1);
$frequency = array_count_values($words);
return $frequency;
}
// Analyze the word count of the text
$wordFrequency = wordCount($text);
// Print the word count
foreach ($wordFrequency as $word => $count) {
echo $word . ": " . $count . "<br>";
}
?>
This program accepts a text, analyzes its word count, and displays the words along with their respective counts. This can be beneficial for performing text analysis tasks.
4. File Upload and Validation
Handling file uploads and validating them is a crucial part of web development. A PHP program that allows users to upload files and applies various validations can enhance your understanding of file handling and data integrity.
Example:
<?php
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$file = $_FILES['file'];
// File validation
if ($file['error'] === UPLOAD_ERR_OK) {
$allowedExtensions = ['jpg', 'jpeg', 'png'];
$maxFileSize = 2 * 1024 * 1024;
$fileName = $file['name'];
$fileSize = $file['size'];
$fileExtension = strtolower(pathinfo($fileName, PATHINFO_EXTENSION));
// Check file extension
if (!in_array($fileExtension, $allowedExtensions)) {
echo "Invalid file extension. Only jpg, jpeg, and png files are allowed.";
}
// Check file size
if ($fileSize > $maxFileSize) {
echo "File size exceeds the limit. Maximum file size allowed is 2MB.";
}
/*
Code for file handling (e.g., moving the file to a specific directory)
*/
echo "File uploaded successfully!";
} else {
echo "Error occurred during file upload.";
}
}
<form method="post" enctype="multipart/form-data">
<input type="file" name="file" required>
<button type="submit">Upload</button>
</form>
?>
This program handles file uploads and performs validations such as checking file extension and size. IT provides feedback to the user based on the validation results.
5. Database Connectivity and CRUD Operations
PHP is often used in conjunction with databases to create dynamic web applications. Understanding how to connect to a database and perform CRUD (Create, Read, Update, Delete) operations is vital for building robust web applications.
Example:
<?php
// Database connection configuration
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$dbname = "your_database";
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Perform CRUD operations
/*
Code for CRUD operations (e.g., inserting, selecting, updating, deleting records)
*/
// Close connection
$conn->close();
?>
This program demonstrates how to establish a connection to a database, perform CRUD operations, and close the connection. IT provides a foundation for building dynamic web applications with PHP and databases.
6. Image Thumbnail Generator
Image processing is a common task in web development. A PHP program that generates image thumbnails can be immensely useful for optimizing Website performance and enhancing user experience.
Example:
<?php
// Original image file
$originalImage = "path/to/original/image.jpg";
// Thumbnail dimensions
$thumbnailWidth = 200;
$thumbnailHeight = 150;
// Load the original image
$image = imagecreatefromjpeg($originalImage);
// Create thumbnail
$thumbnail = imagecreatetruecolor($thumbnailWidth, $thumbnailHeight);
imagecopyresampled($thumbnail, $image, 0, 0, 0, 0, $thumbnailWidth, $thumbnailHeight, imagesx($image), imagesy($image));
// Save the thumbnail
imagejpeg($thumbnail, "path/to/thumbnail/image.jpg");
// Free up memory
imagedestroy($image);
imagedestroy($thumbnail);
?>
This program takes an original image, creates a thumbnail of specified dimensions, and saves IT to the desired location. IT utilizes the GD library for image manipulation.
7. User Authentication System
Building a user authentication system is fundamental for secure web applications. A PHP program that handles user registration, login, and logout functionalities can significantly enhance your understanding of authentication mechanisms.
Example:
<?php
// Registration form handling
if ($_SERVER['REQUEST_METHOD'] === 'POST' && isset($_POST['register'])) {
$username = $_POST['username'];
$password = $_POST['password'];
// Store user credentials in the database
// ...
echo "Registration successful!";
}
// Login form handling
if ($_SERVER['REQUEST_METHOD'] === 'POST' && isset($_POST['login'])) {
$username = $_POST['username'];
$password = $_POST['password'];
// Validate user credentials from the database
// ...
echo "Login successful!";
}
// Logout functionality
if (isset($_GET['logout'])) {
// Perform logout
// ...
echo "Logout successful!";
}
?>
This program handles user registration, login, and logout functionalities. IT showcases the basic flow of user authentication with PHP and provides a starting point to build more complex authentication systems.
Conclusion
These seven PHP programs illustrate the range of possibilities and depth of functionalities that can be achieved using PHP. By exploring and understanding these examples, you can significantly enhance your coding skills and broaden your horizons in PHP development. Remember to experiment, modify, and apply these concepts to real-world projects to further solidify your knowledge and expertise.
FAQs
1. How can I run these PHP programs?
To run these PHP programs, you need to have PHP installed on your local machine or a web server. You can create separate PHP files for each program and execute them by accessing the corresponding URLs in a web browser or by using the PHP command-line interface (CLI).
2. Can I modify these examples to suit my specific needs?
Absolutely! These examples serve as a foundation for your learning and understanding. Feel free to modify and adapt them according to your specific requirements. Experimenting with different scenarios and making changes will deepen your understanding of PHP programming.
3. Are there any specific requirements for using these programs?
Yes, some programs may require additional libraries or extensions to be installed, such as the GD library for image processing. Ensure that you have the necessary dependencies installed before running the programs. Additionally, database-related programs will require a compatible database system like MySQL or PostgreSQL.
4. Can I use these programs in my own projects?
Definitely! These programs are excellent starting points and examples that can be utilized in your projects. They provide a solid foundation for various web development tasks, and by integrating them into your projects, you can save time and streamline your coding process.
Remember to understand the concepts behind each program and adapt them according to your specific project’s requirements and coding standards.