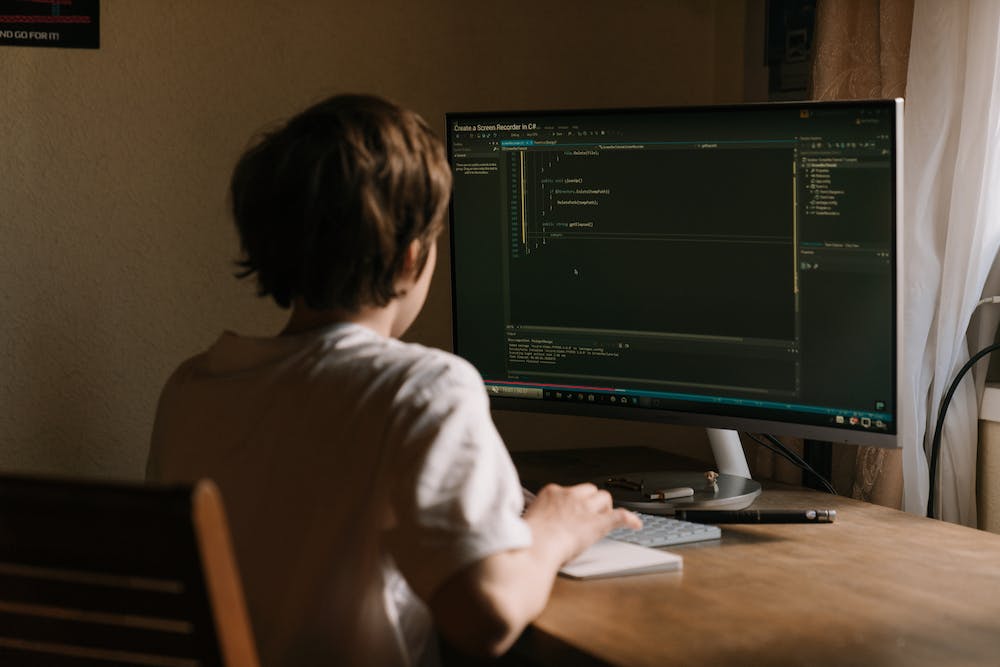
PHP is a versatile and powerful scripting language that is widely used for web development. One of the key features of PHP is the $_POST array, which allows you to collect form data sent by the POST method. However, there are several hacks and best practices that can help you maximize the efficiency of handling $_POST data in your PHP applications. In this article, we will explore 5 secret hacks for making the most out of PHP $_POST, and #4 will certainly blow your mind!
1. Use isset() to Check for Form Submissions
When working with form submissions using PHP $_POST, IT is essential to check if the form has been submitted before processing the data. This can be easily achieved using the isset() function. By using isset() to check if the form fields are set, you can ensure that your code only processes the form data when the form is actually submitted. Here’s an example:
if (isset($_POST['submit'])) {
// Process the form data
}
2. Sanitize $_POST Data to Prevent Security Vulnerabilities
Security should always be a top priority when working with form data in PHP. It is crucial to sanitize the $_POST data to prevent potential security vulnerabilities such as SQL injection and cross-site scripting (XSS) attacks. You can use functions like htmlentities() and mysqli_real_escape_string() to sanitize the $_POST data before using it in your application. Here’s an example:
$username = htmlentities($_POST['username']);
$password = mysqli_real_escape_string($conn, $_POST['password']);
3. Use Extract() to Simplify Access to $_POST Data
Accessing the individual form fields in the $_POST array can sometimes be tedious, especially when dealing with a large number of form fields. To simplify the process, you can use the extract() function to import the form data into the current symbol table, making it easier to access the form fields directly. Here’s an example:
extract($_POST);
echo $username;
echo $email;
4. Utilize Array Functions for Handling Multiple Form Inputs
When working with forms that contain multiple input fields with similar names (e.g., multiple checkbox selections or multiple file uploads), it can be challenging to handle the $_POST data efficiently. In such cases, you can make use of array functions in PHP to simplify the process. For example, you can use functions like array_map() or array_filter() to manipulate and filter the array of form inputs as needed.
5. Improve Performance with Back-End Validation
While client-side validation is essential for providing a seamless user experience, it is equally important to perform back-end validation of the $_POST data to ensure data integrity and consistency. Implementing back-end validation logic can help you catch any potential errors or discrepancies in the form data and provide meaningful feedback to the user. Additionally, back-end validation can prevent unnecessary server-side processing by rejecting invalid or malicious form submissions early in the process.
Conclusion
Maximizing the efficiency of handling PHP $_POST data is essential for building secure and reliable web applications. By applying the secret hacks and best practices outlined in this article, you can streamline the process of collecting, processing, and validating form data, ultimately enhancing the performance and security of your PHP applications. Whether it’s simplifying access to form fields, sanitizing input data, or leveraging array functions, these hacks will undoubtedly take your PHP $_POST efficiency to the next level.
FAQs
Q: Can I use the $_POST array for file uploads?
A: No, the $_POST array is used for collecting form data sent by the POST method. For file uploads, you should use the $_FILES array instead.
Q: Is it necessary to sanitize all form data collected via $_POST?
A: Yes, it is essential to sanitize all form data, including data collected via $_POST, to prevent security vulnerabilities such as SQL injection and XSS attacks.
Q: How can I prevent form resubmissions when using $_POST?
A: To prevent form resubmissions, you can use techniques such as redirect-after-post, PRG (Post/Redirect/Get) pattern, or unique form tokens to ensure that form submissions are not processed more than once.