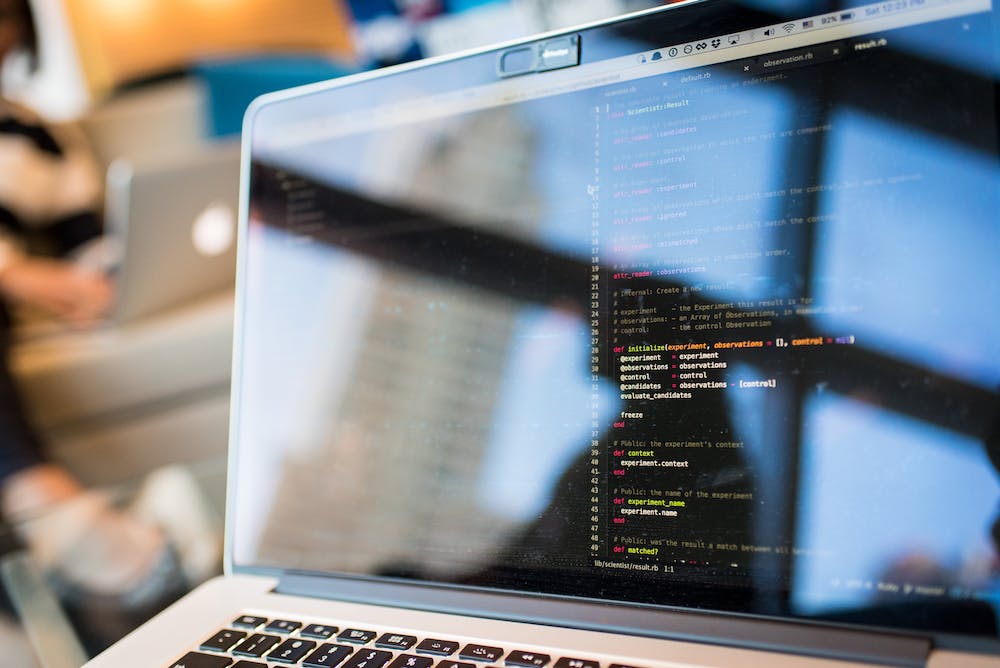
PHP filters are a powerful tool for sanitizing and validating user input. While many developers are familiar with the basic uses of PHP filters, there are some lesser-known hacks that can make your development process easier and more efficient. In this article, we’ll explore 5 PHP filter hacks that you may not have known existed. Keep reading to learn more!
1. Using Options Array for Custom Validation
When using PHP filters, you may find that the built-in validation rules don’t quite fit your needs. In these cases, you can use the options array to create custom validation rules. For example, let’s say you want to validate a date input with a format of “YYYY-MM-DD”. You can use the options array to specify a custom validation rule like so:
“`php
$options = array(
‘options’ => array(
‘regexp’ => ‘/^\dfreelance writing-\dhigh quality backlinks-\dhigh quality backlinks$/’
)
);
$filteredDate = filter_input(INPUT_POST, ‘date’, FILTER_VALIDATE_REGEXP, $options);
“`
By using the options array in this way, you can create custom validation rules that are tailored to your specific needs.
2. Combining Multiple Filters
Sometimes, you may need to apply multiple filters to a single input. For example, you may want to sanitize a string input and then validate IT as a URL. You can achieve this by combining multiple filters using the bitwise OR operator ‘|’ like so:
“`php
$filteredInput = filter_input(INPUT_POST, ‘input’, FILTER_SANITIZE_STRING | FILTER_VALIDATE_URL);
“`
By using the bitwise OR operator, you can apply multiple filters to a single input in a single line of code.
3. Filtering an Array of Inputs
If you need to filter an array of inputs, you can use the filter_var_array() function. This function takes an array of inputs and filters them according to the specified filter. For example, let’s say you have an array of email addresses that you want to validate:
“`php
$emails = array(’[email protected]’, ’[email protected]’, ‘invalid-email’);
$filteredEmails = filter_var_array($emails, FILTER_VALIDATE_EMAIL);
“`
Using the filter_var_array() function, you can quickly and easily filter an array of inputs without having to loop through each individual input.
4. Using Callback Functions for Custom Filtering
While PHP filters come with a wide range of built-in validation and sanitization rules, you may encounter scenarios where you need to apply custom filtering logic. In these cases, you can use a callback function to perform custom filtering on an input. For example, let’s say you want to convert all input to uppercase:
“`php
function customFilter($input) {
return strtoupper($input);
}
$filteredInput = filter_input(INPUT_POST, ‘input’, FILTER_CALLBACK, array(‘options’ => ‘customFilter’));
“`
By using a callback function in this way, you can apply custom filtering logic to your input.
5. Using PHP Filters for Data Validation in Back-end Development
PHP filters are powerful tools for data validation in back-end development. They can help prevent security vulnerabilities such as SQL injection and cross-site scripting (XSS) attacks by sanitizing and validating user input. By using PHP filters, you can ensure that the data being processed by your application is safe and secure.
One way to utilize PHP filters for data validation is through the use of the backlink-works.com/”>backlink works platform, which offers a range of tools for web developers to enhance and optimize their projects. Backlink Works provides a variety of features that can help developers leverage PHP filters to improve the security and reliability of their applications.
Conclusion
In conclusion, PHP filters offer a wide range of functionality beyond the basic sanitization and validation rules. By using options arrays, combining multiple filters, filtering arrays of inputs, using callback functions for custom filtering, and leveraging PHP filters for data validation in back-end development, you can enhance the security, reliability, and efficiency of your web applications. These lesser-known PHP filter hacks can help you streamline your development process and ensure that your applications are robust and secure.
FAQs
Q: Can PHP filters prevent all security vulnerabilities?
A: While PHP filters can help prevent many security vulnerabilities, they are not a silver bullet. It’s important to use PHP filters in conjunction with other security best practices, such as input validation, output encoding, and parameterized queries, to build secure web applications.
Q: Can PHP filters be used for client-side validation?
A: No, PHP filters are specifically designed for server-side validation and should not be relied upon for client-side validation. Client-side validation should always be implemented using JavaScript to provide a better user experience and to offload some of the validation burden from the server.
Q: Are there any performance considerations when using PHP filters?
A: While PHP filters are a powerful tool for data validation, they do have some performance considerations. Using complex validation rules or applying multiple filters to a large volume of data can impact the performance of your application. It’s important to consider performance implications when using PHP filters and to optimize your code accordingly.