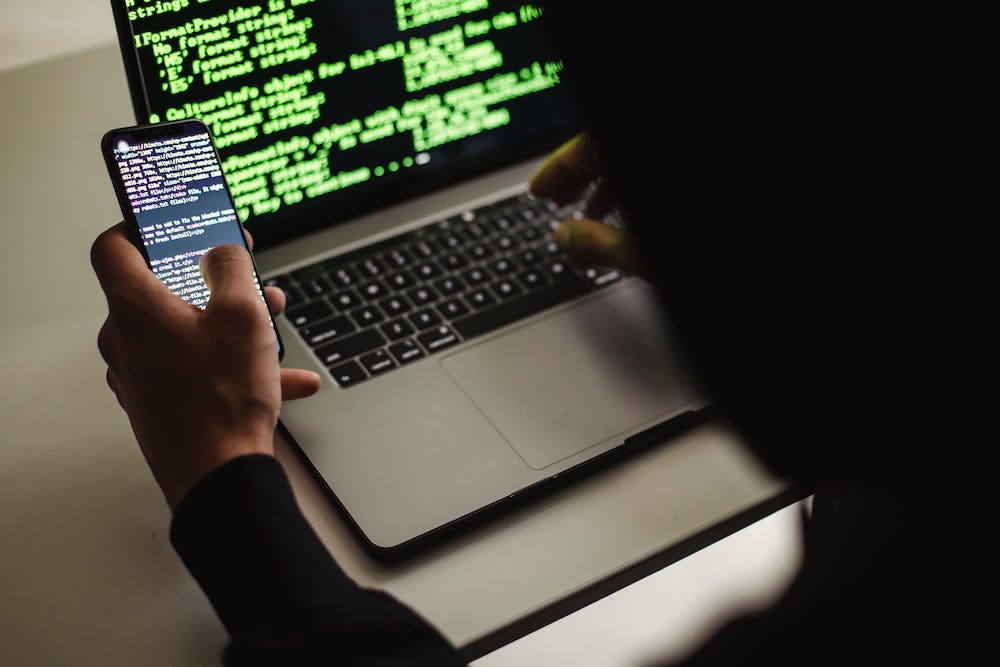
JavaScript is a powerful and versatile programming language that is widely used for creating dynamic and interactive web pages. One of the fundamental features of JavaScript is the ‘if and’ statement, which allows developers to control the flow of their code based on certain conditions. In this article, we will explore five mind-blowing ways to use ‘if and’ statements in JavaScript for maximum code efficiency. These techniques will help you write cleaner, more compact, and more maintainable code, ultimately improving the performance of your web applications.
1. Chained ‘if and’ Statements
Chained ‘if and’ statements are a powerful way to check multiple conditions in a single block of code. Instead of using nested ‘if’ statements, you can chain multiple conditions together using the ‘&&’ operator. This can significantly reduce the complexity of your code and make IT more readable. For example:
if (condition1 && condition2 && condition3) {
// Execute code block if all conditions are true
}
This approach is particularly useful when you have several related conditions that need to be checked together. By chaining the conditions, you can ensure that all of them are satisfied before executing the code block, thus improving the efficiency of your code.
2. ‘if and’ Statements with Ternary Operator
The ternary operator is a powerful shorthand for writing ‘if’ statements in JavaScript. It allows you to write conditional expressions in a more concise and elegant way. By combining ‘if and’ statements with the ternary operator, you can further streamline your code and make it more efficient. Here’s an example:
let result = (condition) ? trueValue : falseValue;
In the above example, the ‘condition’ is evaluated, and if it is true, the ‘trueValue’ is assigned to the ‘result’ variable; otherwise, the ‘falseValue’ is assigned. This approach can be especially useful when you need to make simple decisions based on a single condition, and it can help reduce the number of lines of code in your application.
3. De Morgan’s Laws for ‘if and’ Statements
De Morgan’s Laws provide a powerful way to simplify complex logical expressions in JavaScript. By applying these laws to ‘if and’ statements, you can reduce the number of conditions you need to check and make your code more efficient. De Morgan’s Laws state that the negation of a conjunction (AND) is the disjunction (OR) of the negations of the terms and the negation of a disjunction (OR) is the conjunction (AND) of the negations of the terms. In other words:
// Original statement
if (!(condition1 && condition2)) {
// Execute code block
}
// Equivalent statement using De Morgan's Laws
if (!condition1 || !condition2) {
// Execute code block
}
By applying De Morgan’s Laws, you can simplify complex logical expressions and make them easier to understand and maintain. This can not only improve the efficiency of your code but also reduce the likelihood of logical errors.
4. Short-Circuit Evaluation
JavaScript uses short-circuit evaluation to optimize ‘if and’ statements. Short-circuit evaluation means that the second operand is evaluated only if the first operand does not determine the result. This can be leveraged to write more efficient and concise code. For example:
// Traditional approach
if (condition1) {
if (condition2) {
// Execute code block
}
}
// Short-circuit approach
if (condition1 && condition2) {
// Execute code block
}
By using short-circuit evaluation, you can avoid unnecessary evaluations and improve the performance of your code. This technique is particularly useful when dealing with complex conditions that involve multiple logical operators.
5. ‘if and’ Statements with Function Calls
Another powerful way to use ‘if and’ statements in JavaScript is to combine them with function calls. By encapsulating the logic inside a function, you can make your code more modular and reusable. This can lead to cleaner and more maintainable code. Here’s an example:
function isConditionMet() {
// Perform condition checks
return (condition1 && condition2);
}
if (isConditionMet()) {
// Execute code block
}
By using function calls within ‘if and’ statements, you can encapsulate complex logic and make it more understandable. This approach promotes code reusability and can ultimately lead to more efficient and maintainable code.
Conclusion
In conclusion, JavaScript ‘if and’ statements are a fundamental part of writing efficient and maintainable code. By leveraging the techniques discussed in this article, you can make the most out of ‘if and’ statements and improve the overall efficiency of your code. From chained ‘if and’ statements to short-circuit evaluation, these techniques can help you write cleaner, more concise, and more readable code, ultimately leading to better-performing web applications.
FAQs
Q: How can I decide which technique to use for ‘if and’ statements?
A: The choice of technique for ‘if and’ statements depends on the specific requirements of your application and the complexity of the conditions you need to check. Chained ‘if and’ statements are useful for checking multiple related conditions, while the ternary operator can be used for simple conditional expressions. De Morgan’s Laws can help simplify complex logical expressions, and short-circuit evaluation can optimize performance. Function calls with ‘if and’ statements can promote modularity and reusability.
Q: Can I combine these techniques for maximum code efficiency?
A: Absolutely! You can combine these techniques based on the specific needs of your application to achieve maximum code efficiency. For example, you can use chained ‘if and’ statements with short-circuit evaluation, or combine function calls with De Morgan’s Laws for a more modular and efficient code structure.