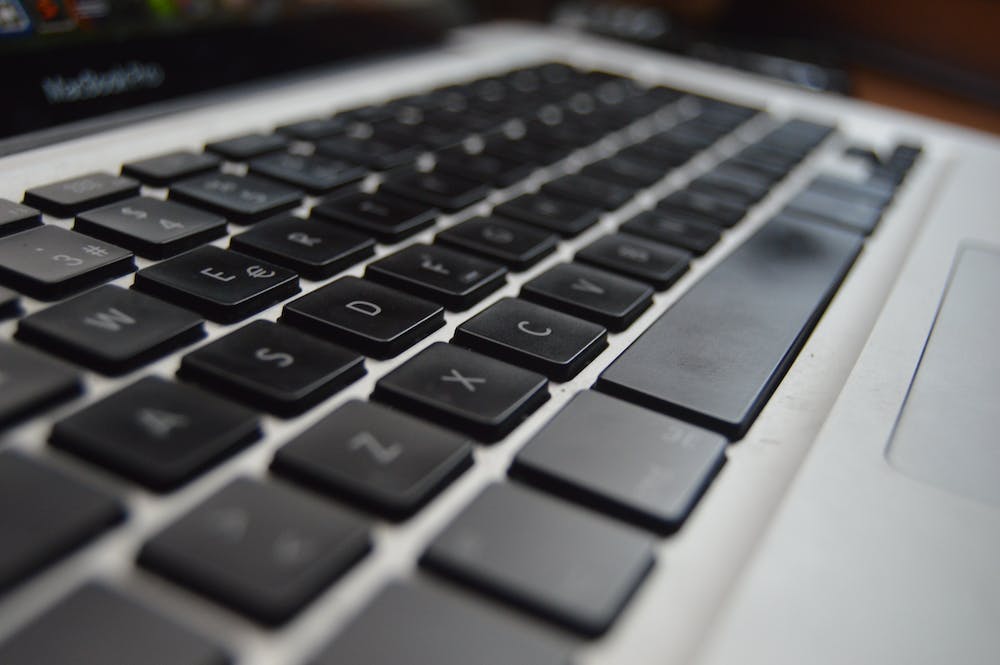
Matplotlib is a powerful and versatile library for creating visualizations in Python. Whether you’re a data scientist, machine learning engineer, or just someone who loves tinkering with code, there are a few Matplotlib code hacks that can take your visualizations to the next level. In this article, we’ll explore five mind-blowing Matplotlib code hacks that you need to try ASAP!
1. Creating Interactive Visualizations with Widgets
Matplotlib can be used to create interactive visualizations with the help of widgets. By using the IPython widgets library, you can add sliders, buttons, and other interactive elements to your plots, allowing users to dynamically change the visualized data. This hack is especially useful for exploring large datasets or presenting data in a more engaging way.
Example:
“`python
import numpy as np
import matplotlib.pyplot as plt
from ipywidgets import interact
x = np.linspace(0, 2 * np.pi, 100)
y = np.sin(x)
@interact(freq=(1, 10, 0.1))
def plot(freq):
plt.plot(x, np.sin(freq * x))
plt.show()
“`
2. Customizing Plot Styles with Seaborn
Seaborn is a popular data visualization library that works seamlessly with Matplotlib. By using Seaborn’s built-in themes and color palettes, you can easily customize the style of your plots to make them more visually appealing. This hack is great for creating professional-looking visualizations with minimal effort.
Example:
“`python
import seaborn as sns
sns.set_style(‘whitegrid’)
sns.set_palette(‘viridis’)
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.show()
“`
3. Animating Plots with FuncAnimation
Matplotlib’s FuncAnimation class allows you to create animated visualizations with just a few lines of code. By updating the plot data in a loop and using FuncAnimation to continually redraw the plot, you can create captivating animations to visualize dynamic processes or changing data over time.
Example:
“`python
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
fig, ax = plt.subplots()
xdata, ydata = [], []
ln, = plt.plot([], [], ‘r’, animated=True)
def init():
ax.set_xlim(0, 2 * np.pi)
ax.set_ylim(-1, 1)
return ln,
def update(frame):
xdata.append(frame)
ydata.append(np.sin(frame))
ln.set_data(xdata, ydata)
return ln,
ani = FuncAnimation(fig, update, frames=np.linspace(0, 2 * np.pi, 128), init_func=init, blit=True)
plt.show()
“`
4. Creating 3D Visualizations with mplot3d
Matplotlib’s mplot3d toolkit allows you to create 3D visualizations with ease. By leveraging the Axes3D class, you can plot three-dimensional data and create stunning visualizations that provide a deeper understanding of complex datasets. This hack is perfect for visualizing spatial data, mathematical functions, and more.
Example:
“`python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection=’3d’)
x = np.random.standard_normal(100)
y = np.random.standard_normal(100)
z = np.random.standard_normal(100)
ax.scatter(x, y, z)
plt.show()
“`
5. Creating Interactive 3D Visualizations with Plotly
Plotly is a powerful visualization library that works well with Matplotlib and offers interactive 3D plotting capabilities. With Plotly, you can create interactive 3D visualizations that can be explored from different angles, zoomed in and out, and provide a more immersive experience for exploring three-dimensional data. This hack is perfect for showcasing 3D data in a dynamic and engaging way.
Example:
“`python
import plotly.graph_objects as go
x, y, z = (1, 2, 3), (4, 5, 6), (7, 8, 9)
fig = go.Figure(data=[go.Scatter3d(x=x, y=y, z=z, mode=’markers’)])
fig.show()
“`
Conclusion
These five mind-blowing Matplotlib code hacks are just the tip of the iceberg when IT comes to pushing the boundaries of data visualization in Python. By leveraging the power of widgets, customizing plot styles with Seaborn, animating plots with FuncAnimation, creating 3D visualizations with mplot3d, and using Plotly for interactive 3D visualizations, you can take your data visualizations to new heights and communicate your insights more effectively.
FAQs
Q: Can these code hacks be used for real-world data visualizations?
A: Absolutely! These code hacks are designed to enhance the visual appeal and interactivity of your data visualizations, making them suitable for real-world applications such as data analysis, presentations, and reports.
Q: Are there any performance considerations when using these code hacks?
A: While these code hacks may introduce some overhead for more complex visualizations, they are designed to provide a significant boost in visual appeal and interactivity, which can outweigh any minor performance impacts.
Q: Can I combine these code hacks for even more powerful visualizations?
A: Absolutely! Feel free to experiment and combine these code hacks to create even more impressive and engaging data visualizations. The possibilities are endless!