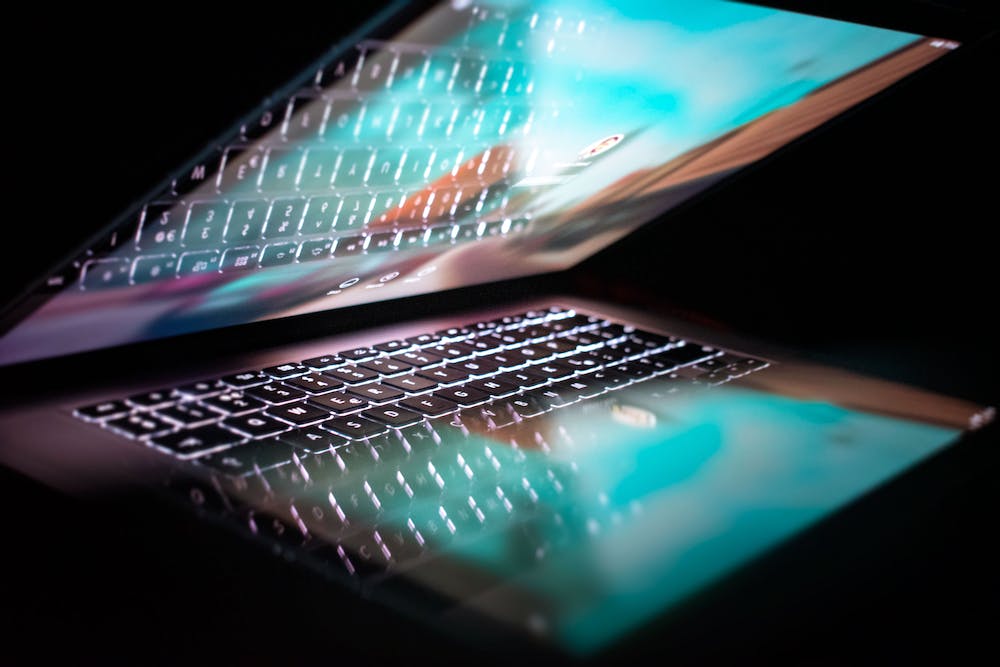
Welcome to our guide on the top 10 Python programs that every beginner should practice. Python is one of the most popular programming languages in the world, known for its simplicity, readability, and flexibility. Whether you’re just starting to learn Python or looking to improve your skills, these programs will help you build a strong foundation and gain valuable experience. Let’s dive in!
1. Hello World
The classic “Hello, World!” program is a must for every beginner. IT‘s a simple program that prints “Hello, World!” to the screen. Here’s the code:
print("Hello, World!")
Run this program to see the famous phrase displayed on your screen.
2. Calculator
Build a basic calculator that takes two numbers as input and performs addition, subtraction, multiplication, and division. This program will help you understand how to take user input and perform mathematical operations using Python.
3. Guess the Number
Create a program that generates a random number and asks the user to guess it. Provide hints such as “Too high” or “Too low” to help the user narrow down their guess. This program will teach you about random number generation and conditional statements in Python.
4. Rock, Paper, Scissors Game
Implement the popular game of rock, paper, scissors in Python. Allow the user to make a choice and compare it with the computer‘s choice to determine the winner. This program will require you to use conditional statements and user input.
5. Palindrome Checker
Write a program that checks whether a given string is a palindrome or not. A palindrome is a word or phrase that reads the same backward as forward. This program will help you practice string manipulation and loops in Python.
6. Fibonacci Sequence
Generate the Fibonacci sequence up to a specified number of terms. The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones. This program will give you a good understanding of loops and basic arithmetic operations in Python.
7. Prime Number Checker
Develop a program that checks whether a given number is prime or not. A prime number is a natural number greater than 1 that has no positive divisors other than 1 and itself. This program will require you to use loops and conditional statements.
8. Reverse a String
Write a program that takes a string as input and returns its reverse. This program will help you practice string manipulation and loops in Python.
9. File Handling
Create a program that reads data from a text file, manipulates it, and writes the modified data back to a new file. This program will teach you about file handling in Python, including reading, writing, and manipulating file data.
10. Simple Web Scraper
Build a basic web scraper that extracts data from a Website. You can start with simple tasks such as fetching the page content or retrieving specific elements from a web page. This program will introduce you to web scraping in Python using libraries like BeautifulSoup or Scrapy.
Conclusion
Congratulations on completing our guide to the top 10 Python programs every beginner should practice. By working through these programs, you’ve gained valuable experience in fundamental Python concepts such as user input, loops, conditional statements, and file handling. These skills will serve as a solid foundation as you continue your journey to become a proficient Python programmer.
FAQs
Q: Are these programs suitable for beginners with no programming experience?
A: Yes, these programs are designed to be beginner-friendly and are a great way to get started with Python programming.
Q: Do I need any special software to run these programs?
A: You can run these programs using a Python IDE such as PyCharm, Jupyter Notebook, or even a simple text editor like Notepad.
Q: Can I modify these programs to add more features?
A: Absolutely! Once you’ve completed the basic versions of these programs, feel free to experiment and expand them with additional features and functionalities.
Q: Where can I find more Python programming resources?
A: There are many great resources available online for learning Python, including tutorials, documentation, and forums. You can also consider enrolling in a Python programming course or joining a coding community for additional support and guidance.
Thank you for reading our guide on the top 10 Python programs every beginner should practice. We hope you’ve found it helpful and inspiring. Happy coding!