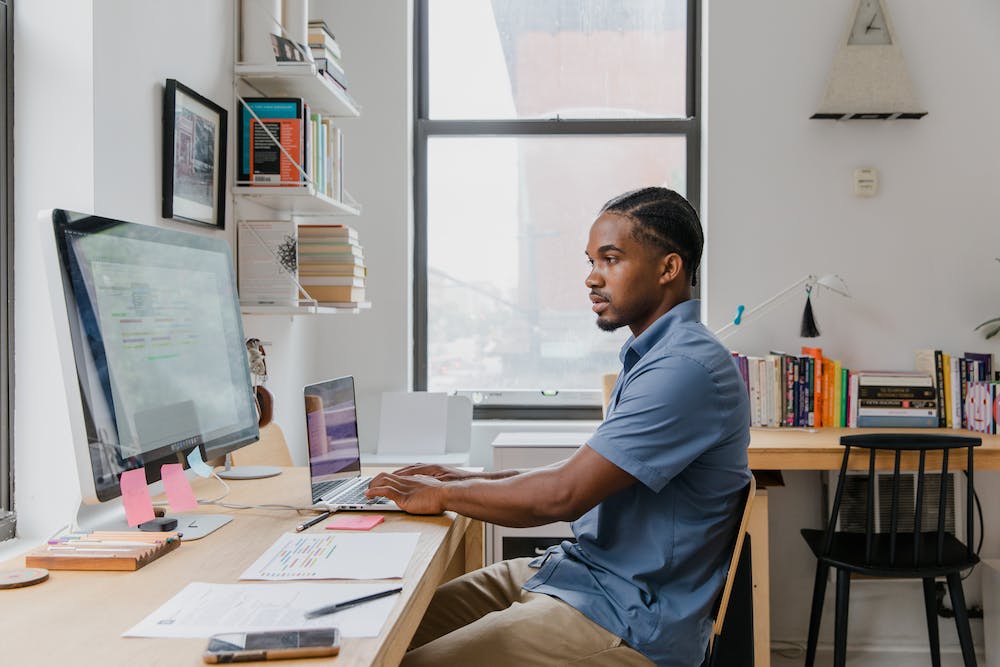
If you are new to the world of programming, Python is an excellent language to start with. IT is easy to learn, versatile, and has a wide range of applications. To help you get started on your coding journey, we have compiled a list of 10 Python practice programs that every beginner should master. By working through these programs, you will not only improve your Python skills but also gain a deeper understanding of programming concepts. So, let’s dive in and start coding like a pro today!
1. Hello World Program
The Hello World program is a classic first program for any beginner learning a new programming language. It serves as a simple introduction to the syntax of the language and helps you understand how to write and run a basic program. In Python, the Hello World program can be written in just one line:
print("Hello, World!")
By running this program, you will see the text “Hello, World!” displayed on your screen, marking the beginning of your Python coding journey.
2. Calculator Program
Building a simple calculator program is a great way to practice using basic arithmetic operators in Python. You can create a program that takes input from the user, performs calculations, and displays the result. For example:
num1 = float(input("Enter the first number: "))
num2 = float(input("Enter the second number: "))
sum = num1 + num2
difference = num1 - num2
product = num1 * num2
quotient = num1 / num2
print("Sum:", sum)
print("Difference:", difference)
print("Product:", product)
print("Quotient:", quotient)
With this program, you can practice taking user input, performing calculations, and displaying the output in Python.
3. Guessing Game Program
A guessing game program is a fun way to practice using conditional statements in Python. In this program, you can ask the user to guess a number and provide feedback based on their input. Here’s an example of a simple guessing game program:
import random
number = random.randint(1, 10)
guess = int(input("Guess the number between 1 and 10: "))
if guess == number:
print("Congratulations! You guessed the correct number.")
else:
print("Sorry, the correct number is", number)
By working on this program, you can practice using the random module and conditional statements in Python.
4. String Manipulation Program
String manipulation is a fundamental skill for any programmer, and Python provides powerful tools for working with strings. You can create a program that takes a string input from the user and performs various operations such as reversing the string, counting the occurrence of a specific character, or converting the case of the string. Here’s an example of a simple string manipulation program:
string = input("Enter a string: ")
print("Reversed string:", string[::-1])
print("Number of characters:", len(string))
print("Uppercase:", string.upper())
print("Lowercase:", string.lower())
By working on this program, you can practice string indexing, string methods, and string formatting in Python.
5. Rock-Paper-Scissors Game Program
The classic game of rock-paper-scissors is a great opportunity to practice using loops and conditional statements in Python. You can create a program that allows the user to play against the computer and keeps track of the score. Here’s an example of a simple rock-paper-scissors game program:
import random
choices = ["rock", "paper", "scissors"]
user_score = 0
computer_score = 0
while True:
user_choice = input("Enter your choice (rock, paper, scissors): ")
computer_choice = random.choice(choices)
if user_choice == computer_choice:
print("It's a tie!")
elif (user_choice == "rock" and computer_choice == "scissors") or
(user_choice == "paper" and computer_choice == "rock") or
(user_choice == "scissors" and computer_choice == "paper"):
print("You win!")
user_score += 1
else:
print("You lose!")
computer_score += 1
play_again = input("Do you want to play again? (yes/no): ")
if play_again.lower() != "yes":
break
print("Final Score - User:", user_score, "Computer:", computer_score)
By working on this program, you can practice using loops, lists, and conditional statements in Python.
6. Number Guessing Game Program
In contrast to the previous game, this program involves creating a number guessing game where the computer generates a random number and the user tries to guess it. Here’s an example of a simple number guessing game program:
import random
number = random.randint(1, 100)
attempts = 0
print("Guess the number between 1 and 100")
while True:
guess = int(input("Enter your guess: "))
attempts += 1
if guess == number:
print("Congratulations! You guessed the correct number in", attempts, "attempts.")
break
elif guess < number:
print("Too low, try again.")
else:
print("Too high, try again.")
By working on this program, you can practice using loops, conditional statements, and the random module in Python.
7. File Management Program
Working with files is a common task in programming, and Python provides powerful tools for file management. You can create a program that reads from or writes to a text file, manipulates the contents, or performs file operations such as renaming or deleting. Here’s an example of a simple file management program:
# Write to a file
with open("example.txt", "w") as file:
file.write("Hello, World!")
# Read from a file
with open("example.txt", "r") as file:
content = file.read()
print("File content:", content)
By working on this program, you can practice file handling, reading and writing files, and performing file operations in Python.
8. Simple Web Scraper Program
Web scraping involves extracting data from websites, and Python provides libraries such as Beautiful Soup and requests to make this task easier. You can create a simple web scraper program that retrieves data from a Website and displays it to the user. Here’s an example of a simple web scraper program:
import requests
from bs4 import BeautifulSoup
url = "https://backlink-works.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
print("Title:", soup.title)
By working on this program, you can practice using libraries, making web requests, and parsing HTML content in Python.
9. Basic Data Analysis Program
Python provides a range of libraries for data analysis and visualization, such as NumPy and Matplotlib. You can create a program that analyzes a simple dataset, calculates statistics, and generates visualizations. Here’s an example of a basic data analysis program:
import numpy as np
import matplotlib.pyplot as plt
data = np.random.rand(100)
print("Mean:", np.mean(data))
print("Standard Deviation:", np.std(data))
plt.hist(data, bins=10)
plt.show()
By working on this program, you can practice using data analysis libraries, manipulating arrays, and creating visualizations in Python.
10. Build a Simple API Program
Finally, creating a simple API program is a great way to practice building and consuming APIs in Python. You can create a basic API that serves data or performs a simple operation, and then write a program that consumes this API and displays the results. Here’s an example of a simple API program:
import requests
url = "https://api.example.com/data"
response = requests.get(url)
if response.status_code == 200:
data = response.json()
print("API Response:", data)
else:
print("Failed to fetch data from the API")
By working on this program, you can practice making API requests, handling responses, and working with JSON data in Python.
Conclusion
Mastering these 10 Python practice programs will not only enhance your coding skills but also build a strong foundation for your programming journey. By working on these programs, you will gain hands-on experience with key Python concepts such as conditional statements, loops, file management, web scraping, data analysis, and API consumption. These programs will also help you develop problem-solving skills and a deeper understanding of how to apply Python in real-world scenarios. So, start coding like a pro today by mastering these essential Python practice programs!
FAQs
1. Why is Python a good language for beginners?
Python is a good language for beginners because of its simple and easy-to-read syntax, extensive libraries and frameworks, and wide range of applications in web development, data analysis, artificial intelligence, and more.
2. How can I improve my Python skills as a beginner?
You can improve your Python skills as a beginner by practicing coding regularly, working on small projects, participating in coding challenges, and learning from online resources and tutorials.
3. What are some key concepts to focus on while learning Python?
Some key concepts to focus on while learning Python include variables and data types, conditional statements and loops, functions and modules, file handling, error handling, and working with libraries and APIs.
4. Are there any online communities for Python beginners?
Yes, there are several online communities for Python beginners, such as the Python subreddit, Python Discord server, and various programming forums and communities where you can connect with other learners and experienced developers.
5. How can I stay motivated while learning Python?
You can stay motivated while learning Python by setting realistic goals, celebrating small victories, seeking support from peers, staying curious and exploring new concepts, and remembering the reasons why you want to learn programming.
6. Is there a recommended IDE for Python beginners?
There are several recommended IDEs for Python beginners, such as PyCharm, Visual Studio Code, and IDLE. It’s important to choose an IDE that you are comfortable with and that suits your specific needs and preferences.
7. How can I get feedback on my Python coding projects?
You can get feedback on your Python coding projects by sharing them with other developers in online communities, participating in code review sessions, seeking mentorship from experienced programmers, and taking part in coding workshops and hackathons.
8. What are some resources for learning Python programming?
There are many resources for learning Python programming, including online courses and tutorials on platforms like Codecademy, Coursera, and Udemy, as well as books, documentation, and official Python resources such as the Python website and Python Package Index (PyPI).
9. Where can I find Python coding challenges and exercises?
You can find Python coding challenges and exercises on websites such as LeetCode, HackerRank, and CodeWars, as well as in Python programming books, online courses with assignments, and coding challenge platforms tailored specifically for Python developers.
10. How can I apply Python programming in real-world projects?
You can apply Python programming in real-world projects by working on small applications, web development projects, data analysis and visualization tasks, automation and scripting, and by contributing to open-source projects on platforms like GitHub and GitLab.
By following this guide and mastering these Python practice programs, you will be well-equipped to start coding like a pro and take your Python skills to the next level. Remember to practice consistently, seek help when needed, and stay curious and passionate about programming. Happy coding!