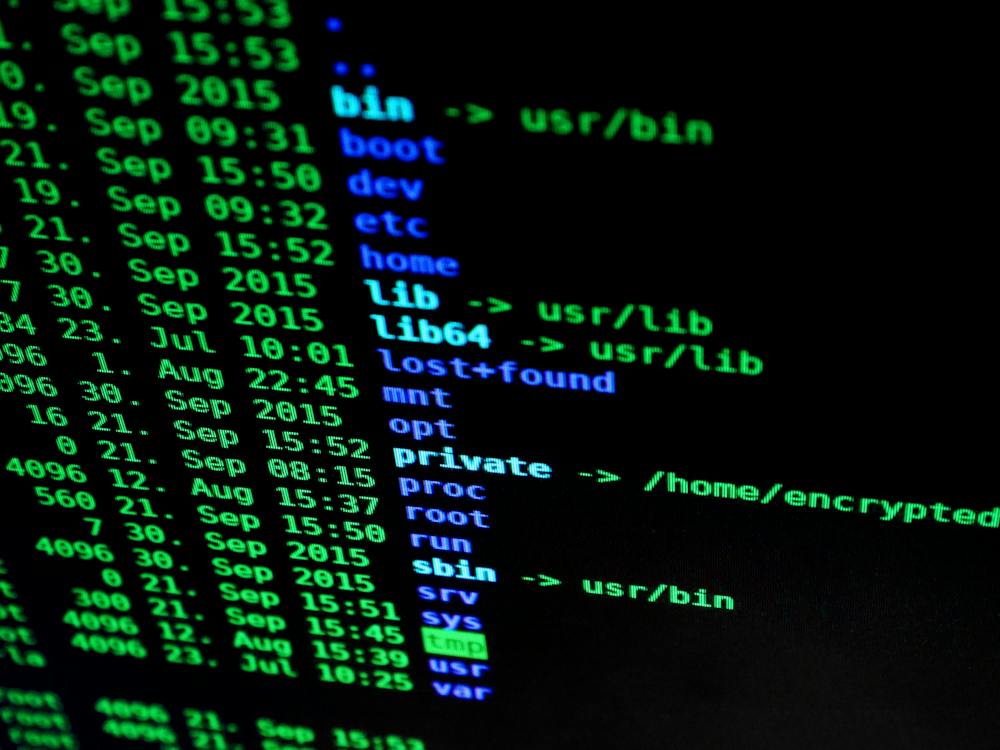
Python is a powerful and versatile programming language that is widely used for a variety of applications, from web development to data analysis. One of the key reasons for its popularity is its readability and clean syntax. However, even experienced Python developers may not be aware of some of the advanced code formatting tricks that can significantly enhance their coding experience. In this article, we will explore 10 Python code formatting tricks that will blow your mind and change the way you write code forever.
#1: Utilize f-strings for String Formatting
String formatting is a common task in Python, and IT‘s important to use the most efficient and readable method. With the introduction of f-strings in Python 3.6, string formatting has become easier and more intuitive than ever. Rather than using the traditional % or .format() methods, f-strings allow you to embed expressions inside string literals, making the code more readable and concise.
For example:
name = 'John'
age = 30
print(f'My name is {name} and I am {age} years old.')
#2: Use PEP 8 for Consistent Code Style
PEP 8 is the official style guide for Python code, and following its recommendations can greatly improve the readability and maintainability of your code. It covers a wide range of topics, from indentation and line length to naming conventions and import statements. Tools like autopep8 can automatically format your code according to PEP 8 standards, ensuring consistent and clean code throughout your projects.
#3: Take Advantage of Type Annotations
Python 3.6 introduced the concept of type annotations, allowing you to specify the types of function arguments and return values. While Python is dynamically typed, type annotations are not enforced at runtime but can be used by static analysis tools like mypy to catch potential errors and improve code readability. By adding type annotations to your code, you can make it more self-documenting and easier for others to understand and maintain.
#4: Embrace Context Managers for Resource Management
Context managers, introduced in Python 2.5, provide a convenient way to manage resources such as files, sockets, and database connections. By using the with statement, you can ensure that the resources are properly cleaned up and released, even in the presence of exceptions. The contextlib module provides tools for creating custom context managers, allowing you to encapsulate resource management logic in a clean and reusable way.
#5: Use List Comprehensions for Concise Iteration
List comprehensions are a powerful and concise way to create lists in Python. They allow you to perform transformations and filtering on iterable data in a single line of code, eliminating the need for verbose for loops and conditional statements. By using list comprehensions, you can write more expressive and readable code while maintaining performance and efficiency.
#6: Leverage Namedtuples for Immutable Data Structures
Namedtuples are a convenient way to create immutable data structures with named fields. They provide a lightweight alternative to defining custom classes for simple data containers, allowing you to access fields by name rather than index. Namedtuples are particularly useful for representing records and data transfer objects, improving the clarity and maintainability of your code.
#7: Use “_” for Unused Variables
In Python, it’s common to use the underscore character (_) as a placeholder for unused variables or function arguments. This convention can help to indicate that a variable is intentionally unused and prevent compiler warnings or linter errors. It also makes the code more readable by signaling to other developers that a certain variable is intended to be ignored.
#8: Avoid Unpacking in Loop Headers
While unpacking in loop headers can be convenient, it can also lead to readability and maintainability issues, especially when dealing with complex data structures. Instead of unpacking values directly in the loop header, consider using unpacking in a separate line, which can make the code more explicit and easier to understand.
#9: Use Decorators for Code Reuse and Modularity
Decorators are a powerful feature in Python that allows you to modify or extend the behavior of functions and methods. By using decorators, you can encapsulate common functionality, such as logging, caching, or authentication, and apply it to multiple functions without duplicating code. Decorators promote code reuse and modularity, making your codebase more maintainable and extensible.
#10: Document Code with Docstrings
Docstrings are a built-in feature of Python that allow you to provide inline documentation for modules, classes, functions, and methods. By using descriptive and informative docstrings, you can improve the readability and maintainability of your code, making it easier for other developers to understand and use your code. Properly documented code is essential for collaborative projects and long-term code maintenance.
Conclusion
These 10 Python code formatting tricks are just a few examples of the many ways you can improve the readability, maintainability, and expressiveness of your Python code. By incorporating these best practices into your coding workflow, you can write cleaner, more efficient code that is easier to understand and maintain. Whether you’re a beginner or an experienced Python developer, taking the time to learn and apply these formatting tricks will undoubtedly have a positive impact on your coding experience.
FAQs
Q: How do I enable PEP 8 auto-formatting in my IDE?
A: Many popular code editors and integrated development environments (IDEs) offer plugins or built-in tools for automatically formatting your code according to PEP 8 standards. For example, in Visual Studio Code, you can install the “Python” extension, which includes support for autopep8 and pylint, enabling you to format and lint your code with a single keystroke.
Q: Are there any performance implications of using list comprehensions?
A: In general, list comprehensions are optimized for performance and are typically faster than equivalent for loop and append operations. However, it’s important to be mindful of memory usage when working with large datasets, as list comprehensions create a new list in memory. If memory usage is a concern, consider using lazy evaluation with generator expressions or other memory-efficient techniques.
Q: Can namedtuples be used as dictionary keys?
A: Yes, namedtuples can be used as dictionary keys, as they are hashable and immutable. This makes them particularly useful for creating lightweight data structures that can be used as keys in dictionaries or elements in sets, providing a convenient way to associate named fields with corresponding values.