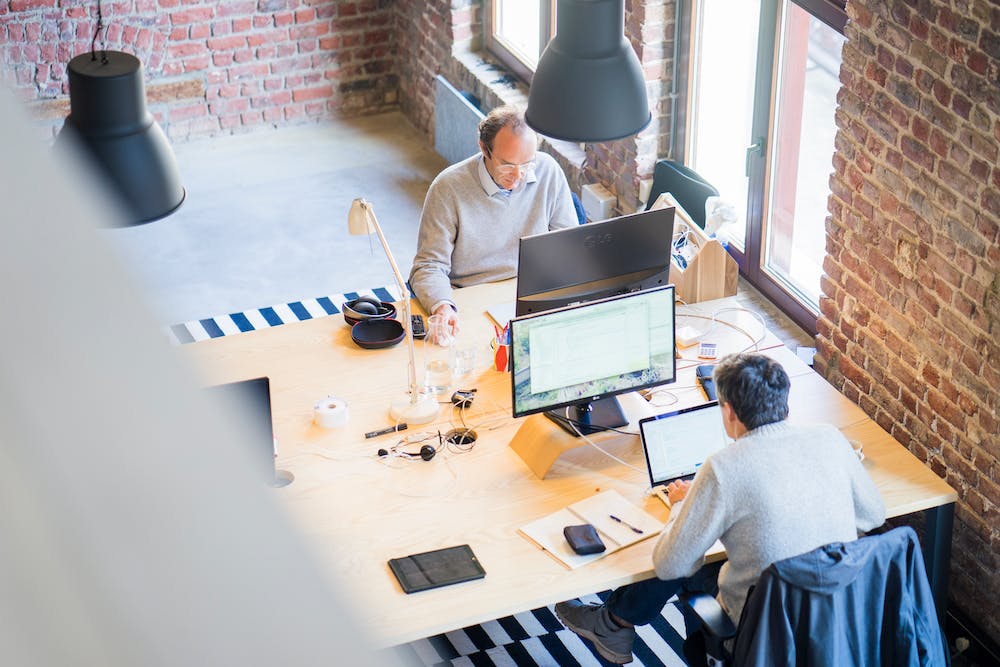
Python is a versatile and popular programming language that can be used for a wide range of applications, including data analysis, web development, and software development. One area where Python excels is in its ability to create pattern programs, which are a series of characters or symbols arranged in a specific shape or design. These patterns can be used for various purposes, such as creating visual designs, formatting output, or solving coding challenges.
1. Print a Simple Star Pattern
One of the simplest pattern programs to create in Python is a star pattern. This can be achieved using nested loops to print a specific number of stars on each line. Here’s an example:
def print_star_pattern(rows):
for i in range(0, rows):
for j in range(0, i + 1):
print("*", end=' ')
print("\r")
2. Print a Number Pattern
Another common pattern is a number pattern, where a series of numbers are printed in a specific shape or sequence. Here’s an example of printing a number pattern in Python:
def print_number_pattern(rows):
num = 1
for i in range(0, rows):
num = 1
for j in range(0, i + 1):
print(num, end=' ')
num += 1
print("\r")
3. Print a Solid Rectangle Pattern
A solid rectangle pattern can be achieved by using nested loops to print a specific number of symbols on each line. Here’s an example of printing a solid rectangle pattern in Python:
def print_solid_rectangle(rows, columns):
for i in range(rows):
for j in range(columns):
print("*", end=' ')
print("\r")
4. Print a Hollow Rectangle Pattern
A hollow rectangle pattern is similar to a solid rectangle pattern, but with spaces inside the rectangle to create the hollow effect. Here’s an example of printing a hollow rectangle pattern in Python:
def print_hollow_rectangle(rows, columns):
for i in range(rows):
for j in range(columns):
if i == 0 or i == rows - 1 or j == 0 or j == columns - 1:
print("*", end=' ')
else:
print(" ", end=' ')
print("\r")
5. Print a Pyramid Pattern
A pyramid pattern can be created by using nested loops to control the number of symbols printed on each line. Here’s an example of printing a pyramid pattern in Python:
def print_pyramid(rows):
for i in range(0, rows):
for j in range(0, rows - i - 1):
print(end=" ")
for j in range(0, i + 1):
print("*", end=" ")
print("\r")
6. Print a Pascal’s Triangle Pattern
Pascal’s triangle is a pattern of numbers that forms a triangular shape. IT‘s created by summing adjacent elements in preceding rows. Here’s an example of printing Pascal’s triangle pattern in Python:
def print_pascals_triangle(rows):
for i in range(rows):
val = 1
for j in range(0, i + 1):
print(val, end=" ")
val = int(val * (i - j) / (j + 1))
print("\r")
7. Print a Floyd’s Triangle Pattern
Floyd’s triangle is a right-angled triangular pattern of natural numbers. It’s created by continuously incrementing a number and arranging it in a right-angled triangular shape. Here’s an example of printing Floyd’s triangle pattern in Python:
def print_floyds_triangle(rows):
num = 1
for i in range(0, rows):
for j in range(0, i + 1):
print(num, end=" ")
num += 1
print("\r")
8. Print a Diamond Pattern
A diamond pattern is a symmetrical shape that forms a diamond-like design. It can be created by using nested loops to control the number of symbols printed on each line. Here’s an example of printing a diamond pattern in Python:
def print_diamond(rows):
for i in range(0, rows):
for j in range(0, rows - i - 1):
print(end=" ")
for j in range(0, i + 1):
print("*", end=" ")
print("\r")
for i in range(rows, 0, -1):
for j in range(0, rows - i + 1):
print(end=" ")
for j in range(0, i - 1):
print("*", end=" ")
print("\r")
9. Print a Hourglass Pattern
An hourglass pattern is a symmetrical shape that forms the design of an hourglass. It can be created by using nested loops to control the number of symbols printed on each line. Here’s an example of printing an hourglass pattern in Python:
def print_hourglass(rows):
for i in range(rows, 0, -1):
for j in range(0, rows - i):
print(end=" ")
for j in range(0, i):
print("*", end=" ")
print("\r")
for i in range(0, rows + 1):
for j in range(0, rows - i):
print(end=" ")
for j in range(0, i):
print("*", end=" ")
print("\r")
10. Print a Cross Pattern
A cross pattern is a simple design of a cross formed by arranging symbols in a specific shape. Here’s an example of printing a cross pattern in Python:
def print_cross(rows):
for i in range(0, rows):
for j in range(0, rows):
if i == j or i + j == rows - 1:
print("*", end=" ")
else:
print(" ", end=" ")
print("\r")
Conclusion
Creating pattern programs in Python is a fun and challenging way to improve your coding skills and enhance your understanding of nested loops and control structures. By mastering these 10 must-know pattern programs, you’ll be well-equipped to tackle a wide range of coding challenges and create visually appealing designs using Python.
FAQs
Q: Are pattern programs important in Python?
A: Pattern programs are an important part of learning Python programming as they can help improve problem-solving skills and strengthen your understanding of control structures and nested loops.
Q: How can I practice creating pattern programs in Python?
A: You can practice creating pattern programs in Python by experimenting with different loop structures and logic to achieve the desired patterns. Additionally, there are many online coding platforms and resources that provide pattern program challenges for practice.
Q: Can pattern programs be used in real-world applications?
A: While pattern programs may seem like simple exercises, they can be applied to real-world applications such as formatting output in text-based applications, creating visual designs in graphical interfaces, and solving coding challenges that involve pattern recognition.
Q: Where can I find more examples of pattern programs in Python?
A: You can find more examples of pattern programs in Python by exploring online coding forums, tutorial websites, and programming communities. Additionally, there are many Python programming books and courses that include pattern program exercises and examples.