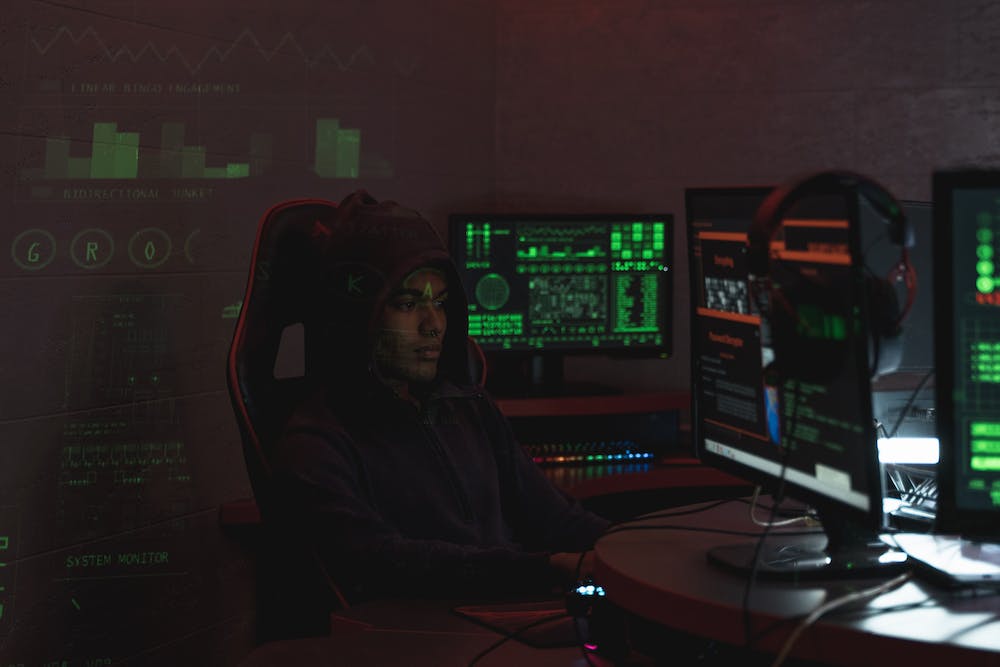
Python is a versatile and powerful programming language that has gained immense popularity among developers. Not only is IT known for its simplicity and readability, but Python also offers numerous features and techniques that make coding more efficient. In this article, we will explore 10 mind-blowing Pythonic code secrets that can truly enhance your programming skills. Brace yourself, because #7 is truly exceptional!
1. List Comprehensions
List comprehensions are an elegant and concise way to create new lists. Instead of writing traditional for loops and appending elements to a list, you can achieve the same result with just one line of code. For example, let’s say you want to create a list containing the squares of numbers from 1 to 10:
squares = [x**2 for x in range(1, 11)]
2. Generator Expressions
Generator expressions are similar to list comprehensions but are more memory-efficient as they produce the elements one-by-one, instead of creating the entire list in memory. This is particularly useful when dealing with large datasets or infinite sequences. To demonstrate this, let’s create a generator expression that yields the Fibonacci sequence:
fib = (fibonacci(x) for x in range(10))
3. Context Managers
Context managers allow you to properly manage resources by defining setup and teardown actions within a single construct. Python’s with
statement makes IT easy to use context managers. For example, if you want to open and read a file, you can use a context manager to automatically close the file when you’re done:
with open('file.txt') as f:
content = f.read()
4. Unpacking
Python’s unpacking feature allows you to assign multiple values to multiple variables in a single line. This can be incredibly useful when dealing with functions that return multiple values or when swapping variable values. For instance:
a, b = 1, 2
5. Decorators
Decorators are a powerful Python concept that allows you to modify the behavior of a function or class without changing their source code. This is achieved by using the @
symbol followed by the decorator name above the function or class definition. For example, you can create a decorator to log the execution time of a function:
import time
def timer_decorator(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print('Execution time:', end_time - start_time)
return result
return wrapper
@timer_decorator
def my_function():
# Function implementation goes here
pass
6. Lambda Functions
Lambda functions, also known as anonymous functions, allow you to create small, one-line functions without assigning them a name. They can be particularly useful when you need to pass a simple function as an argument to another function. For instance, let’s say we want to sort a list of names based on their lengths:
names = ['Alice', 'Bob', 'Charlie', 'Dave']
sorted_names = sorted(names, key=lambda x: len(x))
7. The Walrus Operator
Ah, here’s the mind-blowing Pythonic code secret you’ve been waiting for! Introduced in Python 3.8, the walrus operator (:=
) allows you to assign a value to a variable as part of an expression. This can be particularly useful in while loops or conditional statements. Let’s consider an example where we want to read lines from a file until we reach an empty line:
with open('file.txt') as f:
while (line := f.readline().strip()) != '':
print(line)
8. Underscore as a Variable Name
In Python, you can use an underscore (_
) as a variable name to indicate a “throwaway” or unused variable. This can be helpful when you want to ignore a particular value returned by a function or during iteration. For example:
for _ in range(10):
# Perform some action
pass
9. Defaultdict
The defaultdict
class from Python’s collections module allows you to define a default value for nonexistent keys in a dictionary. This eliminates the need for manual checks and avoids KeyError
exceptions. Here’s an example:
from collections import defaultdict
my_dict = defaultdict(int)
my_dict['x'] += 1
print(my_dict['x']) # Output: 1
print(my_dict['y']) # Output: 0 (default value)
10. The else
Clause in Loops
In Python, loops can have an else
clause that is executed when the loop has exhausted all iterations. This can be useful when you want to perform some action only if the loop completed without encountering a break
statement. For example, let’s find if a number is prime:
num = 17
for i in range(2, int(num**0.5) + 1):
if num % i == 0:
print(num, 'is not prime')
break
else:
print(num, 'is prime')
Conclusion
Python offers a plethora of code secrets and techniques that can greatly enhance your programming skills. The ten secrets we revealed in this article provide a glimpse into the world of Pythonic code. From list comprehensions and generators to context managers and decorators, these techniques can help you write more efficient, elegant, and concise code.
FAQs
Q: Are these Pythonic code secrets suitable for beginners?
A: While some of the techniques may require a basic understanding of Python, they can be beneficial to all skill levels. Beginners can gradually incorporate these secrets into their code as they advance their programming knowledge.
Q: Where can I learn more about Pythonic code?
A: There are numerous online resources, tutorials, and books available that delve into advanced Python concepts and best practices. Additionally, actively participating in the Python community, such as joining forums or attending meetups, can provide valuable insights into Pythonic code.
Q: Can I use these techniques in other programming languages?
A: While some of the concepts discussed in this article are specific to Python, many ideas can be applied to other programming languages. However, syntax and implementation may vary, so IT‘s essential to consult the documentation or resources specific to the language you’re working with.
Q: Will using these Pythonic code secrets improve my code performance?
A: While these secrets can improve code readability and conciseness, their impact on performance may vary depending on the specific use case. IT‘s always recommended to benchmark and profile your code to identify any performance bottlenecks.
Remember, code secrets are meant to enhance your programming skills and improve the quality of your code. Experiment with these techniques, adapt them to your projects, and continue exploring the vast capabilities of Python!