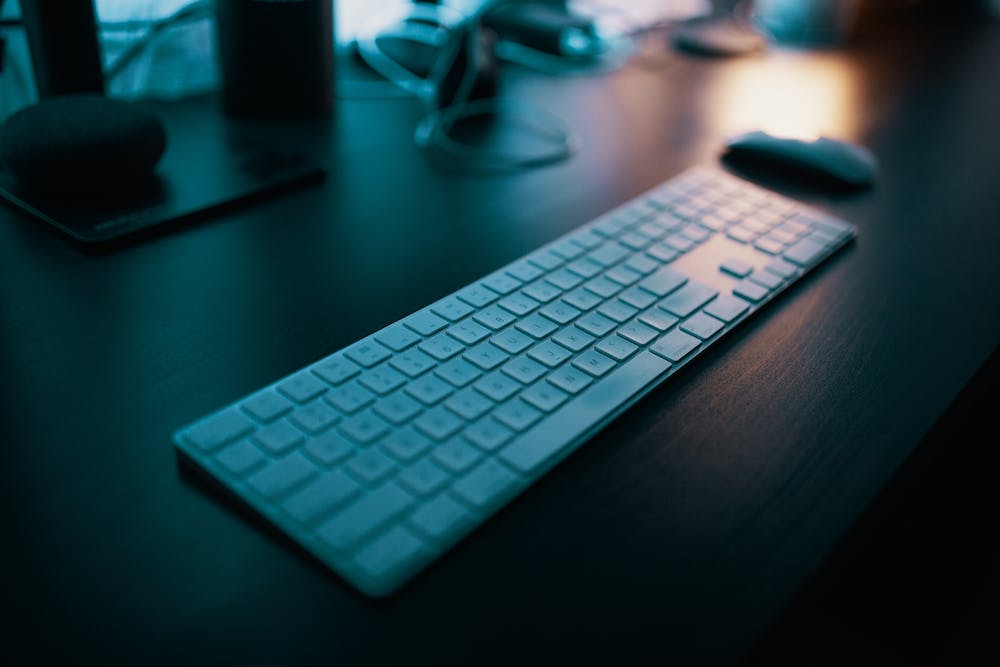
Introduction
Python is a popular programming language known for its simplicity and readability. IT is widely used in various fields such as web development, data science, and artificial intelligence. One of the most exciting aspects of Python is its turtle module, which allows us to create amazing graphics and animations with just a few lines of code. In this article, we will explore 10 mind-blowing Python turtle codes that will leave you awestruck.
1. Spiral – The Classic
The first code we will explore is creating a spiral pattern using turtle graphics. We start by importing the turtle module and creating a turtle object. Then, by using a loop and the turtle’s forward and right methods, we can create a spiral effect.
import turtle
my_turtle = turtle.Turtle()
my_turtle.speed(10)
for i in range(200):
my_turtle.forward(i)
my_turtle.right(91)
2. Square Spiral
Another fascinating code is creating a square spiral using turtle graphics. Similar to the previous code, we start by importing the turtle module and creating a turtle object. Then, using a loop and some calculations, we can draw a square spiral.
import turtle
my_turtle = turtle.Turtle()
my_turtle.speed(10)
length = 10
angle = 90
for _ in range(100):
my_turtle.forward(length)
my_turtle.right(angle)
length += 10
3. Square Maze
Creating a square maze using turtle graphics is a fun challenge. In this code, we utilize loops and turtle movements to create an intricate maze-like structure. We can customize the maze size and complexity as per our requirements.
import turtle
my_turtle = turtle.Turtle()
my_turtle.speed(10)
length = 10
angle = 90
for _ in range(100):
my_turtle.forward(length)
my_turtle.right(angle)
length += 10
4. Tree
Tree structures are mesmerizing to see, and with turtle graphics, we can create beautiful tree-like patterns. By utilizing recursion and turtle movements, we can draw intricate trees with branches of varying sizes. Here’s an example code:
import turtle
def draw_tree(branch_length, t):
if branch_length < 5:
return
else:
t.forward(branch_length)
t.right(20)
draw_tree(branch_length - 10, t)
t.left(40)
draw_tree(branch_length - 10, t)
t.right(20)
t.backward(branch_length)
my_turtle = turtle.Turtle()
my_turtle.speed(100)
my_turtle.left(90)
my_turtle.up()
my_turtle.backward(200)
my_turtle.down()
draw_tree(100, my_turtle)
5. Spirograph
Spirograph patterns are visually stunning and can be easily created using turtle graphics. By combining circular movements and rotations, we can generate unique and mesmerizing designs. Here’s a sample code that creates a simple spirograph pattern:
import turtle
import math
def draw_spirograph(radius, color):
my_turtle = turtle.Turtle()
my_turtle.speed(10)
my_turtle.color(color)
for angle in range(0, 360, 10):
x = math.radians(angle)
y = radius * math.cos(x)
my_turtle.goto(int(y), int(radius * math.sin(x)))
draw_spirograph(100, "blue")
6. Fractal Koch Snowflake
Koch snowflake is a classic fractal pattern that can be created using turtle graphics. By recursively dividing and drawing lines, we can generate intricate snowflake designs. Here’s an example code:
import turtle
def draw_koch(snowflake_length, levels):
if levels == 0:
turtle.forward(snowflake_length)
else:
draw_koch(snowflake_length / 3, levels - 1)
turtle.left(60)
draw_koch(snowflake_length / 3, levels - 1)
turtle.right(120)
draw_koch(snowflake_length / 3, levels - 1)
turtle.left(60)
draw_koch(snowflake_length / 3, levels - 1)
my_turtle = turtle.Turtle()
my_turtle.speed(100)
my_turtle.up()
my_turtle.backward(200)
my_turtle.down()
draw_koch(300, 4)
7. Flower
A flower created using turtle graphics adds a touch of beauty to any project. By using loops and rotations, we can draw petals to form a stunning flower. Here’s an example code:
import turtle
def draw_flower(petal_length, petal_count):
angle = 360 / petal_count
my_turtle = turtle.Turtle()
my_turtle.speed(10)
for _ in range(petal_count):
my_turtle.forward(petal_length)
my_turtle.backward(petal_length)
my_turtle.right(angle)
draw_flower(100, 6)
8. Heart
Hearts are symbols of love and warmth. Using turtle graphics, we can create a heart shape that can be used in various projects. Here’s a sample code to draw a heart:
import turtle
def draw_heart():
my_turtle = turtle.Turtle()
my_turtle.speed(10)
my_turtle.begin_fill()
my_turtle.left(140)
my_turtle.forward(180)
my_turtle.circle(-90, 200)
my_turtle.right(120)
my_turtle.circle(-90, 200)
my_turtle.forward(180)
my_turtle.end_fill()
draw_heart()
9. Spiral Galaxy
Creating a spiral galaxy using turtle graphics can be a fascinating project. By combining circular movements and rotations, we can generate a mesmerizing galaxy-like design. Here’s an example code:
import turtle
def draw_galaxy():
my_turtle = turtle.Turtle()
my_turtle.speed(10)
for _ in range(180):
my_turtle.forward(200)
my_turtle.right(30)
my_turtle.forward(20)
my_turtle.left(60)
my_turtle.forward(50)
my_turtle.right(30)
draw_galaxy()
10. Rainbow Circle
A vibrant rainbow circle created using turtle graphics can be visually appealing. By utilizing loops, rotations, and different colors, we can form an attractive rainbow pattern. Here’s an example code:
import turtle
def draw_rainbow_circle():
colors = ["red", "orange", "yellow", "green", "blue", "indigo", "violet"]
my_turtle = turtle.Turtle()
my_turtle.speed(10)
my_turtle.width(10)
for i in range(360):
my_turtle.color(colors[i % 7])
my_turtle.forward(1)
my_turtle.right(1)
draw_rainbow_circle()
Conclusion
Python turtle graphics provide an exciting way to explore creativity and art through the use of code. The above examples highlight just a fraction of what can be achieved with this powerful module. By leveraging loops, recursion, and different turtle movements, you can create stunning patterns and designs that are sure to leave you awestruck.
FAQs
Q1: What is Python turtle?
Python turtle is a built-in module in Python that allows the user to create graphics and animations using a turtle object. IT provides a simplified interface for creating amazing visuals with just a few lines of code.
Q2: Can I use turtle graphics for game development?
While turtle graphics are more commonly used for creating visual art, simple games can also be built using the turtle module. However, for more complex games, other libraries and frameworks might be more suitable.
Q3: Are there any resources I can refer to for further learning?
Absolutely! There are plenty of resources available to help you learn more about Python turtle graphics. Some recommended resources include online tutorials, documentation, and books on Python graphics programming.
Q4: Can I customize the turtle object’s appearance?
Yes, you can customize various attributes of the turtle object, such as its shape, color, and speed. This allows you to create unique visuals according to your preferences.
Q5: Can I create my own turtle graphics functions?
Definitely! Python turtle allows you to define your own functions to create custom graphics and animations. This way, you can encapsulate complex patterns or repetitive code into reusable functions to make your code more organized and efficient.
Q6: Are there any performance considerations when using turtle graphics?
Python turtle graphics are great for learning and experimenting; however, they might not be the most performant solution for large-scale projects. If performance is a concern, you might want to consider using other libraries or frameworks tailored specifically for graphics programming.
Q7: Can I export turtle graphics as images or videos?
Yes, IT is possible to export turtle graphics as images or even record them as videos. By using additional Python libraries, such as Pillow or OpenCV, you can save your turtle graphics as images or create videos from frames captured during the animation.