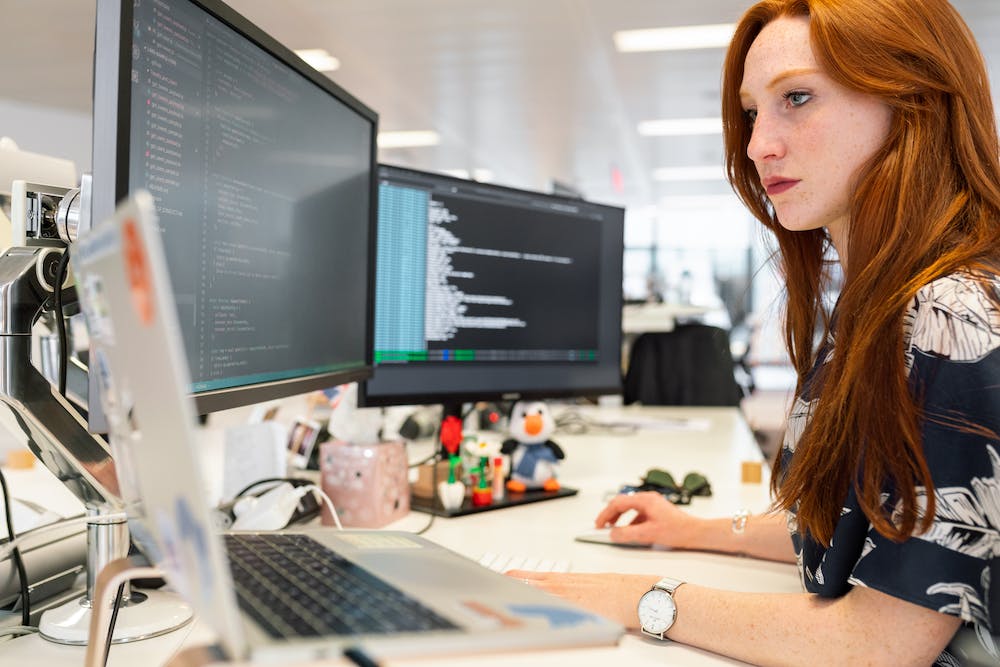
Python is an immensely popular programming language known for its simplicity and versatility. IT offers numerous libraries and modules which makes IT easier to develop applications. However, IT is always beneficial to know some lesser-known time code tricks to enhance your Python coding skills and save time. In this article, we will explore 10 mind-blowing Python time code tricks that you may have never known about!
Have you ever wondered how to measure the execution time of your Python code? Well, Python provides a built-in module called “time” which includes various functions for time-related operations. One of these functions is time.time()
, which returns the current time in seconds since the epoch. By measuring the execution time before and after your code block, you can calculate the duration of code execution.
“`
import time
start_time = time.time()
# Your code here
end_time = time.time()
execution_time = end_time – start_time
print(f”Execution time: {execution_time} seconds”)
“`
Formatting time output is often necessary in applications where the output needs to be in a specific format, such as displaying time in hours, minutes, and seconds. Python’s time module provides the time.strftime()
function to format time according to specific directives. For example, let’s say you want to display the current time in the format “HH:MM:SS”.
“`
import time
current_time = time.strftime(“%H:%M:%S”)
print(current_time)
“`
Python’s time.sleep()
function is quite handy when you need to pause the execution of your code for a specified number of seconds. This can be helpful in situations where you want to introduce a delay between certain operations or simulate real-time behavior in your programs.
“`
import time
print(“Starting…”)
time.sleep(5) # Pause execution for 5 seconds
print(“Resumed…”)
“`
Creating a timing decorator allows you to easily measure the execution time of any function in your code. This can be particularly useful when you are refactoring or optimizing your code. With the help of Python’s time
module, you can easily create a decorator to wrap around your functions.
“`
import time
def timing_decorator(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
execution_time = end_time – start_time
print(f”Execution time: {execution_time} seconds”)
return result
return wrapper
@timing_decorator
def some_function():
# Your code here
pass
some_function()
“`
Converting timestamps from one format to another is a common requirement when working with time-related data. Python’s datetime
module provides useful functions to accomplish this task easily. For instance, let’s say you have a timestamp in UNIX format and you want to convert IT to a string representation of time.
“`
import datetime
timestamp = 1625850000 # UNIX timestamp
converted_time = datetime.datetime.utcfromtimestamp(timestamp).strftime(“%Y-%m-%d %H:%M:%S”)
print(converted_time)
“`
Python’s datetime
module also allows you to determine the day of the week for a given date. By using the datetime.datetime.strptime()
function, you can parse a string representation of a date into a datetime object. Then, you can use the datetime.strftime()
function along with the “%A” directive to obtain the full weekday name.
“`
import datetime
date_string = “2022-07-10”
date_object = datetime.datetime.strptime(date_string, “%Y-%m-%d”)
weekday = date_object.strftime(“%A”)
print(weekday)
“`
Epoch time, also known as UNIX time, represents the number of seconds that have elapsed since January 1, 1970 UTC. Python’s time
module provides easy ways to manipulate and work with epoch time. For example, if you want to get the current epoch time, you can use time.time()
function.
“`
import time
current_epoch_time = int(time.time())
print(current_epoch_time)
“`
Dealing with timezones can be a complex and tricky task. However, Python’s pytz
library makes IT easier by providing timezone database functionality. You can convert a datetime object from one timezone to another using the pytz.timezone()
and astimezone()
methods.
“`
import datetime
import pytz
# Current datetime object with local timezone
current_datetime = datetime.datetime.now()
# Converting to a specific timezone
new_timezone = pytz.timezone(“America/New_York”)
converted_datetime = current_datetime.astimezone(new_timezone)
print(converted_datetime)
“`
Python’s context managers are a powerful way to manage resources and perform actions before and after a piece of code. By creating a timer context manager, you can conveniently measure the execution time of a block of code using Python’s time
module.
“`
import time
from contextlib import contextmanager
@contextmanager
def timer():
start_time = time.time()
yield
end_time = time.time()
execution_time = end_time – start_time
print(f”Execution time: {execution_time} seconds”)
with timer():
# Your code here
pass
“`
If you need to perform timeit measurements, Python provides a built-in module called timeit
. IT allows you to easily compare the performance of different code snippets or functions. You can use timeit.timeit()
to run a specific piece of code multiple times and measure its execution time.
“`
import timeit
code_snippet = ”’
# Your code here
”’
execution_time = timeit.timeit(code_snippet, number=10000)
print(f”Average execution time per loop: {execution_time / 10000} seconds”)
“`
Conclusion
In this article, we explored 10 mind-blowing Python time code tricks that may have remained unknown to you. These tricks can help you measure code execution time, format time output, create timing decorators, convert timestamps, determine the day of the week, work with epoch time and timezones, utilize timer context managers, and leverage the timeit module for performance measurements. By incorporating these tricks into your Python projects, you can enhance your coding efficiency and accomplish time-related tasks more effectively.
FAQs
1. How can I measure the execution time of my Python code?
You can measure the execution time of your Python code by using the time.time()
function from the built-in time module. By recording the start and end times of your code block, you can calculate the duration of code execution.
2. Is there a way to introduce delays in Python code?
Yes, Python provides the time.sleep()
function which allows you to pause code execution for a specific number of seconds. This can be useful in cases where you want to introduce delays between operations or simulate real-time behavior.
3. How can I convert a timestamp to a readable date and time format?
You can convert a timestamp to a readable date and time format using Python’s datetime
module. By utilizing the datetime.datetime.utcfromtimestamp()
function, you can convert a UNIX timestamp into a datetime object. From there, you can format the datetime object using the strftime()
function.
4. What is epoch time and how can I work with IT in Python?
Epoch time, also known as UNIX time, represents the number of seconds that have elapsed since January 1, 1970 UTC. In Python, you can easily obtain the current epoch time using time.time()
function. Additionally, you can manipulate and convert epoch time to various formats using Python’s time module.
5. How can I perform timeit measurements in Python?
To perform timeit measurements in Python, you can utilize the built-in timeit
module. IT allows you to compare the performance of different code snippets or functions by running them multiple times and measuring their execution time using the timeit.timeit()
function.