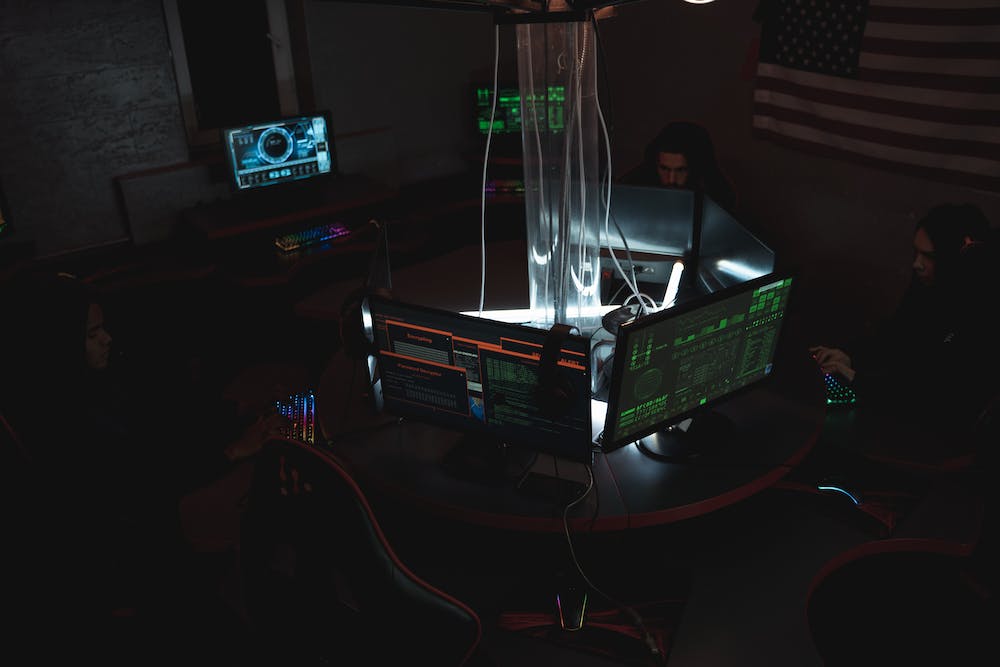
JavaScript is a powerful language that allows you to create dynamic and interactive websites. With its flexibility and versatility, there are numerous ways you can write code to achieve the same functionality. In this article, we will explore 10 mind-blowing JS code hacks that you’ve probably never seen before. These hacks will not only impress you but also change the way you code forever!
-
Dynamic HTML content with InnerHTML Property
The innerHTML property allows you to dynamically change the content of an HTML element. Instead of using document.createElement and appendChild to add content to an element, you can simply assign the new HTML content to the innerHTML property. This makes IT incredibly easy to update the content of an element without having to manipulate the DOM directly.
Example:
< pre >
< script >
document.getElementById('targetElement').innerHTML = '< h1 >New Heading< /h1 >';
< /script >
< /pre >
-
Creating a Range Slider with Input Event
The input event is triggered when the value of an input element changes. You can use this event to create a range slider that dynamically updates its value as the user drags the slider. This provides a smooth and responsive user experience without needing to use the change event, which only triggers when the user releases the mouse button.
Example:
< pre >
< script >
document.getElementById('rangeSlider').addEventListener('input', function() {
document.getElementById('sliderValue').innerHTML = this.value;
});
< /script >
< /pre >
-
Debouncing Input with Timeout
When dealing with user input in forms, you may want to debounce the input to reduce the number of times a function is called. You can achieve this by using the setTimeout function to delay the execution of the function until the user has stopped typing for a certain period of time. This prevents the function from being called repeatedly as the user types.
Example:
< pre >
< script >
let timeout;
document.getElementById('searchInput').addEventListener('input', function() {
clearTimeout(timeout);
timeout = setTimeout(function() {
// Call search function
}, 500);
});
< /script >
< /pre >
-
Creating a Modal with ES6 Template Literals
ES6 introduced template literals, which allow you to create multi-line strings and embed expressions within them. This makes it easy to create dynamic content for a modal without having to concatenate strings or use complex HTML manipulation. Template literals provide a clean and concise way to define the content of a modal.
Example:
< pre >
< script >
const modalContent = `
< div class="modal">
< h2 >Modal Title< /h2 >
< p >Modal Content< /p >
< /div >
`;
document.body.insertAdjacentHTML('beforeend', modalContent);
< /script >
< /pre >
-
Filtering Arrays with Array.filter()
The Array.filter() method allows you to create a new array with all elements that pass a certain condition. This is a powerful tool for filtering data in an array without needing to use a for loop or other manual iteration methods. The concise and expressive syntax of Array.filter() makes it a great addition to your coding arsenal.
Example:
< pre >
< script >
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(num => num % 2 === 0);
console.log(evenNumbers); // Output: [2, 4]
< /script >
< /pre >
-
Chaining Array Methods for Complex Operations
JavaScript arrays have a variety of built-in methods that allow you to perform complex operations in a concise and readable manner. You can chain these methods together to create powerful and expressive code that performs multiple operations on an array in a single line. This makes your code more efficient and easier to understand.
Example:
< pre >
< script >
const numbers = [1, 2, 3, 4, 5];
const result = numbers
.filter(num => num % 2 === 0)
.map(num => num * 2)
.reduce((acc, cur) => acc + cur, 0);
console.log(result); // Output: 12
< /script >
< /pre >
-
Implementing Throttling for Rate-Limiting
Throttling is a technique used to limit the rate at which a function is called. This is particularly useful for handling events that may be triggered frequently, such as scroll or resize events. By implementing throttling, you can ensure that the function is only called at a certain interval, reducing the strain on the browser and improving performance.
Example:
< pre >
< script >
let throttleTimeout;
window.addEventListener('scroll', function() {
if (!throttleTimeout) {
throttleTimeout = setTimeout(function() {
// Call scroll function
throttleTimeout = null;
}, 200);
}
});
< /script >
< /pre >
-
Using Intersection Observer for Lazy Loading
The Intersection Observer API allows you to efficiently observe when an element enters or exits the browser’s viewport. This can be used for lazy loading images, where the image is only loaded when it becomes visible to the user. By utilizing the Intersection Observer, you can improve the performance of your Website by reducing the initial load time of large or numerous images.
Example:
< pre >
< script >
const observer = new IntersectionObserver(entries => {
entries.forEach(entry => {
if (entry.isIntersecting) {
// Load image
observer.unobserve(entry.target);
}
});
});
document.querySelectorAll('img').forEach(img => {
observer.observe(img);
});
< /script >
< /pre >
-
Generating Unique IDs with UUID
Generating unique identifiers can be a challenge, especially when dealing with large datasets or dynamically created elements. The UUID (Universally Unique Identifier) library provides a simple and effective way to generate unique IDs that are virtually guaranteed to be unique. This can be incredibly useful for indexing, key generation, and other data management tasks.
Example:
< pre >
< script src="https://cdnjs.cloudflare.com/ajax/libs/uuid/8.3.2/uuid.min.js" >< /script >
< script >
const uniqueID = uuid.v4();
console.log(uniqueID); // Output: e4eaaaf2-d142-11e1-b3e4-080027620cdd
< /script >
< /pre >
-
Implementing Lazy Initialization with Proxy
The Proxy object enables you to create a placeholder for another object and intercept operations such as property lookup, assignment, enumeration, and function invocation. This can be used for lazy initialization, where the actual object is only created when it is first accessed. Lazy initialization with Proxy can improve the performance of your application by deferring the creation of the object until it is actually needed.
Example:
< pre >
< script >
const lazyInit = new Proxy({}, {
get: function(target, prop) {
if (!target[prop]) {
// Create object
target[prop] = /* Object creation */;
}
return target[prop];
}
});
Conclusion
These 10 mind-blowing JS code hacks demonstrate the power and flexibility of JavaScript as a language. By incorporating these hacks into your coding repertoire, you can streamline your development process, improve the performance of your applications, and create a more engaging user experience. Whether you’re a beginner or an experienced developer, these hacks will undoubtedly change the way you code forever.
FAQs
Q: Can these JavaScript hacks be used in any type of web development?
A: Absolutely! These JavaScript hacks are versatile and can be applied to various types of web development projects, including front-end development, back-end development, and full-stack development.
Q: Are these JavaScript hacks compatible with all web browsers?
A: While most modern web browsers support the latest JavaScript features, it’s always a good practice to check for compatibility and provide fallbacks for older browsers when using advanced JavaScript hacks.
Q: Where can I learn more about JavaScript coding tricks and techniques?
A: You can explore online resources, such as coding forums, tutorials, and online courses, to expand your knowledge of JavaScript coding tricks and techniques. Additionally, you can join coding communities and engage with fellow developers to learn from their experiences and insights.
Q: How can I stay updated on the latest JavaScript trends and best practices?
A: To stay updated on the latest JavaScript trends and best practices, you can subscribe to coding newsletters, follow influential developers and organizations on social media, and attend web development conferences and workshops. backlink works also offers valuable resources and insights on JavaScript coding best practices.