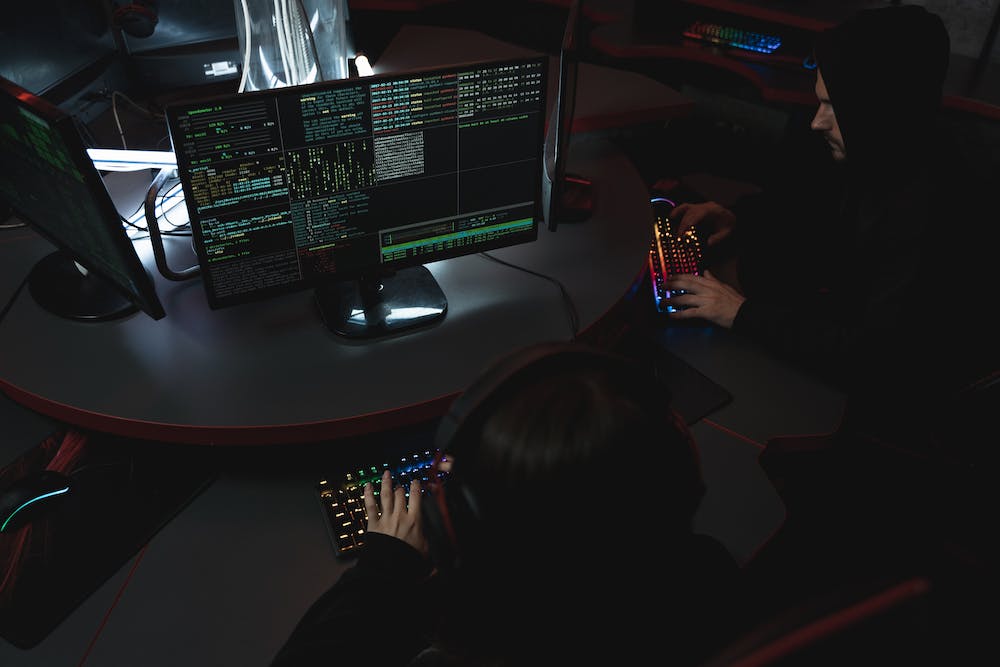
In today’s digital world, web development is a crucial aspect of any business. PHP and MySQL are two of the most popular technologies used for web development. PHP is a server-side scripting language that is used to develop dynamic web pages, while MySQL is a relational database management system that is used to store and manage data.
When IT comes to building web applications, integrating PHP with MySQL is essential. It allows developers to create dynamic, data-driven websites that are both powerful and flexible. In this article, we will discuss 10 essential tips for effective PHP and MySQL integration to help you build robust and efficient web applications.
1. Use Prepared Statements
Prepared statements are a way of writing SQL queries that allows the database to parse and compile the query just once, and then execute it with different parameters multiple times. This can help improve performance and prevent SQL injection attacks. Here’s an example of using prepared statements in PHP:
$stmt = $pdo->prepare('SELECT * FROM users WHERE username = :username');
$stmt->execute(['username' => $username]);
2. Sanitize User Input
When working with user input, it’s important to sanitize the data to prevent SQL injection and other security vulnerabilities. Use PHP’s filter_var()
function to sanitize user input:
$username = filter_var($_POST['username'], FILTER_SANITIZE_STRING);
3. Use Indexing in MySQL
Indexing can significantly improve the performance of MySQL queries, especially for large datasets. Identify the columns that are frequently used in the WHERE clause or JOIN conditions, and create indexes on those columns. For example:
CREATE INDEX index_name
ON table_name (column1, column2, ...);
4. Limit the Data Returned
When fetching data from the database, it’s important to limit the amount of data returned. Use the LIMIT
clause in your SQL queries to restrict the number of rows returned. This can improve performance and reduce memory usage.
5. Optimize Database Queries
Optimizing database queries is crucial for improving the performance of your web application. Use EXPLAIN to analyze your queries and identify any potential bottlenecks. Consider using JOINs, subqueries, and aggregate functions wisely to improve query performance.
6. Use Transactions
Transactions ensure the atomicity, consistency, isolation, and durability (ACID) properties of the database. Use transactions when performing multiple SQL statements to ensure that either all statements are executed, or none are. This can help maintain data integrity and prevent data corruption.
7. Validate and Escaping Form Data
When working with form data, it’s important to validate and escape the input to prevent security vulnerabilities. Use PHP’s htmlspecialchars()
function to escape HTML characters and prevent cross-site scripting (XSS) attacks.
8. Use Stored Procedures
Stored procedures can help improve the security and performance of your web application. They allow you to encapsulate complex SQL logic into a reusable and secure module that can be called from your PHP code.
9. Monitor and Optimize Server Performance
Regularly monitor and optimize your server performance to ensure that your web application is running smoothly. Consider using tools like MySQL’s Performance Schema and PHP’s Xdebug to identify and resolve performance issues.
10. Use ORM Frameworks
Object-relational mapping (ORM) frameworks like Doctrine and Eloquent can simplify the interaction between PHP and MySQL. They provide a higher level of abstraction for database operations and can help improve code maintainability and scalability.
Conclusion
Effective integration of PHP and MySQL is crucial for building robust and efficient web applications. By following the 10 essential tips discussed in this article, you can optimize the performance, security, and maintainability of your web application. From using prepared statements and sanitizing user input to optimizing database queries and utilizing ORM frameworks, these tips will help you build scalable and reliable web applications.
FAQs
Q: Why is PHP and MySQL integration important?
A: PHP and MySQL integration is important for building dynamic and data-driven web applications. PHP provides the server-side scripting language, while MySQL offers a powerful and reliable database management system. Integrating the two technologies allows developers to create robust and efficient web applications.
Q: How can I improve the performance of my PHP and MySQL integration?
A: You can improve the performance of your PHP and MySQL integration by using prepared statements, sanitizing user input, using indexing in MySQL, limiting the data returned, optimizing database queries, and using transactions. Additionally, monitoring and optimizing server performance, using stored procedures, and leveraging ORM frameworks can also help improve performance.
Q: Are there any security considerations when integrating PHP and MySQL?
A: Yes, security is a critical consideration when integrating PHP and MySQL. It’s important to sanitize user input, validate and escape form data, and use prepared statements to prevent SQL injection and other security vulnerabilities. Additionally, using stored procedures and ORM frameworks can help improve the security of your web applications.