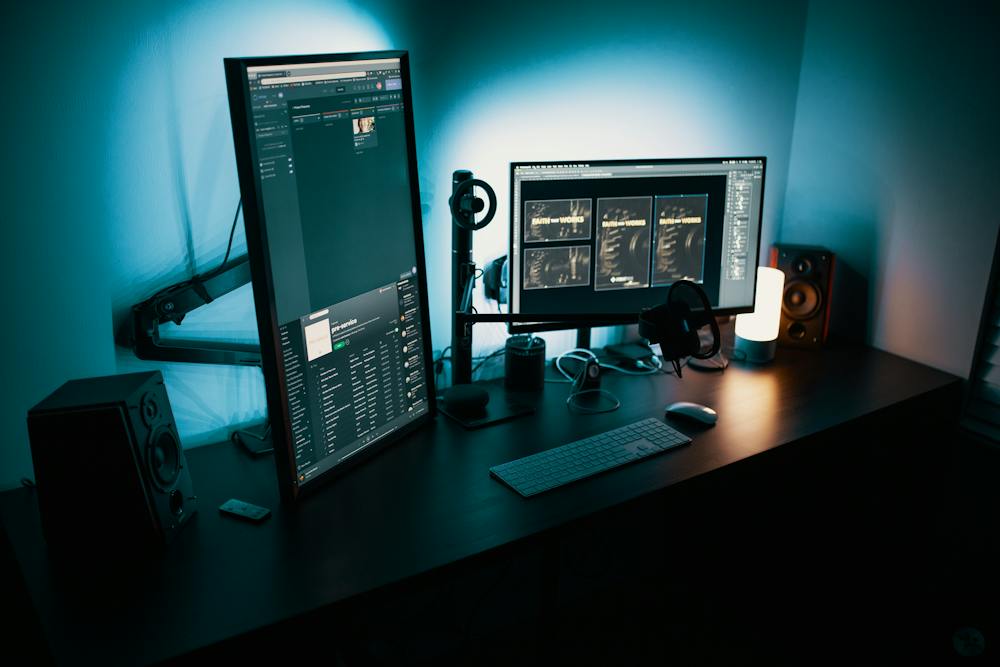
JavaScript is a versatile and powerful language that is used extensively for web development. However, writing clean and maintainable JavaScript code can be a challenge for many developers. In this article, we will discuss 10 essential tips for writing clean JavaScript code that is easy to understand, maintain, and debug.
1. Use Descriptive Variable and Function Names
One of the most important aspects of writing clean JavaScript code is using descriptive variable and function names. Descriptive names make the code more readable and understandable for other developers. For example, instead of using single-letter variable names like “x” or “y”, use meaningful names like “width” and “height”. This not only makes the code easier to understand but also reduces the need for excessive comments.
2. Follow Consistent Coding Conventions
Consistent coding conventions make the code more predictable and easier to read. Choose a coding style and stick to IT throughout your project. This includes indentation, spacing, and naming conventions. Consistency in coding conventions helps in maintaining a clean and organized codebase.
3. Avoid Global Variables
Global variables can lead to naming conflicts and make the code difficult to maintain and debug. Minimize the use of global variables and instead use local variables within functions whenever possible. This ensures that variables are only accessible within their relevant scope, leading to cleaner and more maintainable code.
4. Modularize Your Code
Divide your code into small, reusable modules with well-defined responsibilities. This not only promotes code reusability but also makes it easier to test and maintain. By breaking down your code into smaller modules, you can isolate and fix issues more effectively, leading to cleaner and more robust code.
5. Avoid Nesting Too Many Callbacks
Nesting too many callbacks can lead to a phenomenon known as “callback hell” where the code becomes difficult to read and understand. Use Promises or async/await to handle asynchronous operations in a more readable and manageable way. This not only reduces callback nesting but also makes the code more maintainable and error-resistant.
6. Write Self-Explanatory Code
Write code that is self-explanatory by breaking down complex logic into smaller, well-named functions. Each function should have a single responsibility and be easy to understand without the need for excessive comments. Self-explanatory code reduces cognitive load and makes the codebase more maintainable and scalable.
7. Optimize Loops and Iterations
When working with loops and iterations, optimize your code for performance and readability. Use efficient looping techniques like for loops or Array methods (e.g. map, filter, reduce) instead of nested loops. This not only improves the performance of your code but also makes it more readable and maintainable.
8. Handle Errors Gracefully
Ensure that your code handles errors gracefully by using try-catch blocks or error handling mechanisms. Avoid silent errors that can go unnoticed and lead to unexpected behavior in your application. Properly handling errors makes the code more robust and prevents potential issues from escalating into major problems.
9. Comment Thoughtfully
Although the goal is to write self-explanatory code, there are situations where comments are necessary to clarify complex logic or provide context for future developers. When adding comments, ensure they are meaningful, concise, and relevant to the code they are explaining. Over-commenting can be as detrimental as under-commenting, so strike a balance.
10. Use Linters and Code Formatters
Linters and code formatters help in enforcing coding standards and identifying potential issues in your code. Tools like ESLint, JSHint, and Prettier can be integrated into your development workflow to automatically enforce coding conventions, catch errors, and format your code. This not only helps in maintaining a consistent codebase but also improves the overall quality of your JavaScript code.
Conclusion
Writing clean and maintainable JavaScript code is crucial for the success of any web development project. By following the 10 essential tips discussed in this article – using descriptive names, consistent coding conventions, avoiding global variables, modularizing code, handling callbacks, writing self-explanatory code, optimizing loops, handling errors, commenting thoughtfully, and utilizing linters and code formatters – developers can ensure that their JavaScript code is clean, readable, and easy to maintain. By incorporating these best practices into their workflow, developers can create high-quality JavaScript code that is scalable, maintainable, and robust.
FAQs
Q: Why is writing clean JavaScript code important?
A: Writing clean JavaScript code is important because it improves code readability, maintainability, and scalability. Clean code is easier to understand, debug, and modify, leading to a more efficient development process and a more reliable application.
Q: How can I enforce coding standards in my JavaScript code?
A: You can enforce coding standards in your JavaScript code by using linters and code formatters. These tools can be integrated into your development workflow to automatically identify and fix coding issues, enforce coding conventions, and format your code according to predefined rules.
Q: What are some common mistakes to avoid when writing JavaScript code?
A: Some common mistakes to avoid when writing JavaScript code include using vague variable names, nesting too many callbacks, relying on global variables, neglecting error handling, and overusing comments. By being mindful of these common pitfalls, developers can write cleaner and more maintainable JavaScript code.