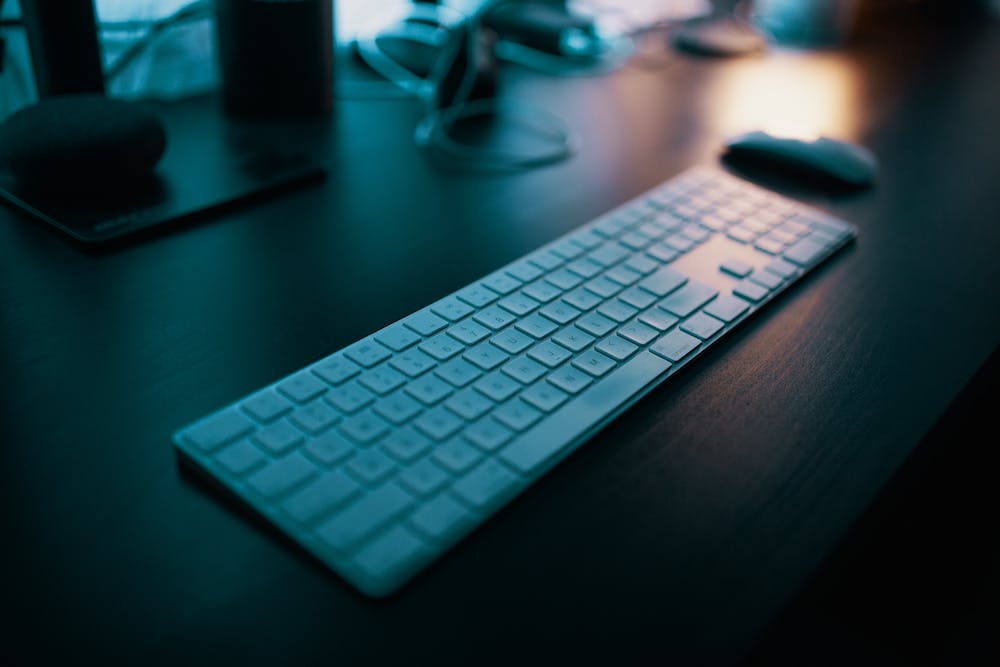
Python is a versatile and powerful programming language that is widely used for various purposes such as web development, data analysis, machine learning, and artificial intelligence. If you are a beginner in Python, IT is essential to learn and understand the fundamental code examples that form the building blocks of the language. In this article, we will walk you through ten essential Python code examples for beginners, explaining their purpose and providing explanations along the way.
Example 1: Hello, World!
Every programming language tutorial starts with a “Hello, World!” example, and Python is no exception. Open your Python editor and enter the following code:
print("Hello, World!")
When you run this code, IT will display “Hello, World!” in the console or the output screen. Congratulations, you have just written your first Python program!
Example 2: Variables and Data Types
In Python, you can store data in variables. Here’s an example:
name = "John"
age = 30
height = 1.75
is_student = True
This code demonstrates how to assign values to variables. “name” is a string variable, “age” is an integer variable, “height” is a float variable, and “is_student” is a boolean variable.
Example 3: Arithmetic Operations
You can perform arithmetic operations in Python. Here are some examples:
num1 = 10
num2 = 5
addition = num1 + num2
subtraction = num1 - num2
multiplication = num1 * num2
division = num1 / num2
remainder = num1 % num2
The above code illustrates how to perform addition, subtraction, multiplication, division, and modulo operations.
Example 4: Lists
A list is a versatile data type that can store multiple values in a single variable. Here’s an example of how to create and manipulate lists:
fruits = ["apple", "banana", "orange"]
print(fruits[0]) # Output: apple
fruits.append("grape")
print(fruits) # Output: ["apple", "banana", "orange", "grape"]
fruits.remove("banana")
print(fruits) # Output: ["apple", "orange", "grape"]
Lists are enclosed in square brackets, and individual elements can be accessed using index values.
Example 5: Decision-Making with if-else
Python provides the if-else construct to make decisions based on certain conditions. Here’s an example:
age = 18
if age >= 18:
print("You are an adult.")
else:
print("You are a minor.")
This code checks the value of “age” and prints a message accordingly.
Example 6: Looping with for and while
Loops allow you to repeat a block of code multiple times. Python supports both for and while loops. Here’s an example:
# for loop
fruits = ["apple", "banana", "orange"]
for fruit in fruits:
print(fruit)
# while loop
counter = 1
while counter <= 5:
print(counter)
counter += 1
The for loop iterates over each element in the list “fruits,” printing each fruit name. The while loop prints the numbers 1 to 5 by incrementing the counter variable.
Example 7: Functions
Functions enable you to encapsulate a block of code that can be reused multiple times. Here’s an example:
def greet(name):
print("Hello, " + name + "!")
greet("John")
greet("Emma")
This code defines a function called “greet” that takes a parameter called “name.” IT prints a personalized greeting message with the name provided.
Example 8: File Handling
Python allows you to perform file operations easily. Here’s an example:
file = open("example.txt", "w")
file.write("Hello, World!")
file.close()
This code opens a file called “example.txt” in write mode and writes the text “Hello, World!” into IT. Finally, IT closes the file.
Example 9: Exception Handling
try:
x = 10 / 0
except ZeroDivisionError:
print("Oops! Division by zero is not allowed.")
This code demonstrates how to handle exceptions using the try-except block. If a zero division error occurs, IT will print a custom error message.
Example 10: Object-Oriented Programming
class Car:
def __init__(self, brand):
self.brand = brand
def drive(self):
print("Driving a " + self.brand + " car.")
my_car = Car("Tesla")
my_car.drive()
This example shows how to define a class and create an object from IT. The “Car” class has a constructor and a method called “drive” that displays a message.
FAQs
1. What can I do with Python?
Python can be used for various purposes, including web development, data analysis, machine learning, and artificial intelligence.
2. Why should I learn Python?
Python is one of the most popular programming languages due to its simplicity, readability, and versatility. IT has a vast community and extensive support for various libraries and frameworks.
3. Can I write Python code in any text editor?
Yes, you can write Python code in any text editor. However, using a dedicated Python editor or integrated development environment (IDE) provides additional features and makes development easier.
4. How can I run a Python program?
To run a Python program, save the code with a .py extension and execute IT using the Python interpreter or through an integrated development environment (IDE).
5. Is Python free?
Yes, Python is an open-source programming language and is free to use.
Learning these ten essential Python code examples will provide a strong foundation for your programming journey. However, Python is a vast language with much more to explore. Keep practicing, experimenting, and diving deeper to become proficient in Python programming.