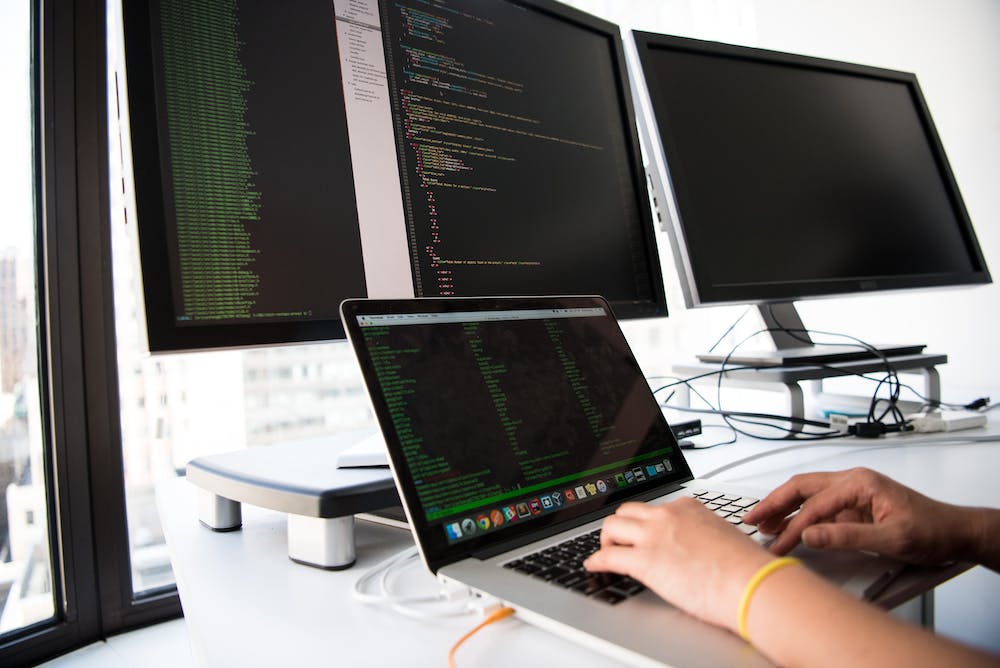
Introduction
PHP (Hypertext Preprocessor) is one of the most widely used server-side scripting languages for web development. IT has a wide range of functions that can simplify and enhance the development process. In this article, we will discuss 10 essential PHP functions that every developer should know. These functions will help you save time and write more efficient and effective PHP code.
1. echo()
The echo() function is used to output text or variables to the browser. IT is often used to display dynamic content on a webpage. Here’s an example:
echo "Hello, World!";
2. isset()
The isset() function is used to determine if a variable is set and not null. IT returns true if the variable is set and false if IT is not set or null. This is often used to check if a form field has been filled out before processing the data. Here’s an example:
if(isset($_POST['name'])) {
echo "Name: ".$_POST['name'];
}
3. strlen()
The strlen() function is used to get the length of a string. IT returns the number of characters in the string. This can be useful for validating input string lengths. Here’s an example:
$name = "John Doe";
echo strlen($name); // Output: 8
4. strtolower()
The strtolower() function is used to convert a string to lowercase. This can be helpful for case-insensitive comparisons or when manipulating string data. Here’s an example:
$text = "Hello, World!";
echo strtolower($text); // Output: hello, world!
5. array()
The array() function is used to create an array. An array is a collection of values that can be accessed by index. Arrays are commonly used to store and manipulate sets of related data. Here’s an example:
$fruits = array("apple", "banana", "orange");
echo $fruits[0]; // Output: apple
6. count()
The count() function is used to get the number of elements in an array. IT returns the array’s length. This can be useful for iterating over arrays or checking if an array is empty. Here’s an example:
$fruits = array("apple", "banana", "orange");
echo count($fruits); // Output: 3
7. include()
The include() function is used to include and execute the contents of an external PHP file. This can be helpful for including reusable code or modularizing your PHP codebase. Here’s an example:
include "header.php";
8. file_get_contents()
The file_get_contents() function is used to read the contents of a file into a string. IT can be used to retrieve the content of a URL or read data from a local file. Here’s an example:
$content = file_get_contents("https://example.com");
echo $content;
9. mail()
The mail() function is used to send an email. IT takes several parameters, such as the recipient’s email address, subject, and body. This can be helpful for sending automated emails or user notifications. Here’s an example:
$to = "[email protected]";
$subject = "Hello";
$message = "This is a test email.";
mail($to, $subject, $message);
10. date()
The date() function is used to format a date and time. IT takes a format string as a parameter and returns the formatted date and/or time. This can be useful for displaying timestamps or formatting dates for specific locales. Here’s an example:
echo date("Y-m-d H:i:s"); // Output: 2022-01-01 12:00:00
Conclusion
These 10 essential PHP functions are just a glimpse of the vast capabilities of PHP. By mastering these functions, developers can significantly improve their ability to create dynamic and efficient web applications. Whether IT‘s handling user input, manipulating strings, or working with arrays, these functions will streamline your PHP development. Keep exploring the PHP documentation to discover more functions and expand your programming horizons.
FAQs
Q: Can I use these functions in other programming languages?
A: No, these functions are specific to PHP and cannot be directly used in other programming languages. However, other languages may offer similar functions with different names or syntax.
Q: Are these functions compatible with all PHP versions?
A: Yes, these functions are part of the core PHP language and are supported in all PHP versions. However, IT‘s always a good practice to check the PHP documentation for any changes or deprecations in newer versions.
Q: How can I learn more PHP functions?
A: The best way to learn more about PHP functions is by exploring the official PHP documentation. IT provides detailed explanations, examples, and usage guidelines for every built-in PHP function. Additionally, there are numerous online tutorials and courses dedicated to PHP development that cover a wide range of functions and topics.
Q: Can I create my own custom functions in PHP?
A: Absolutely! PHP allows developers to define their own custom functions to encapsulate reusable blocks of code. This helps in enhancing code modularity and maintainability.