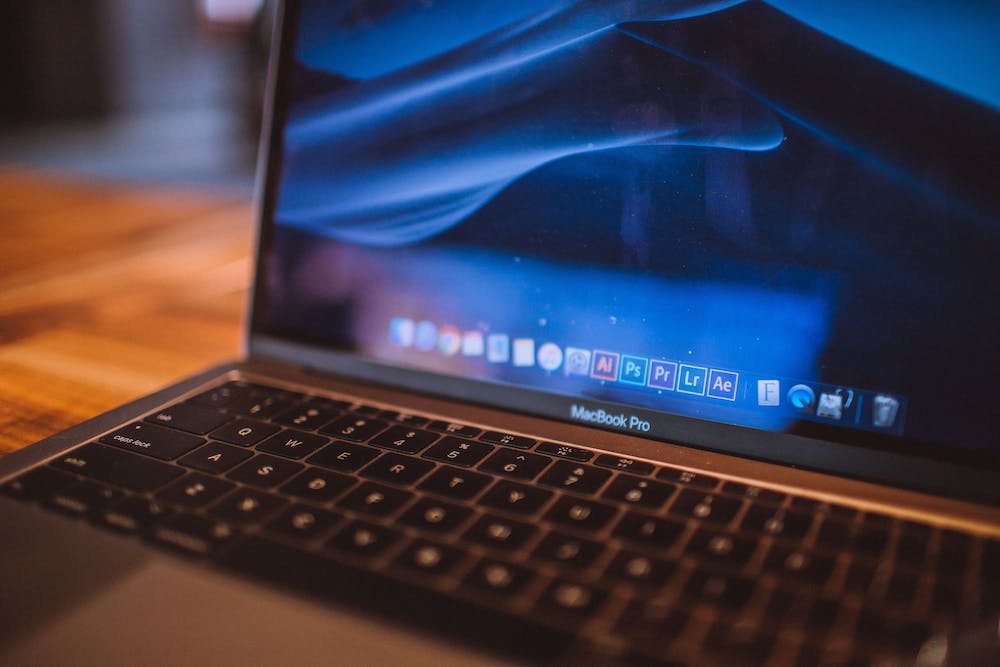
// First PHP Code Snippet
echo “Hello, world!”;
?>
// Looping through an Array using foreach loop
$fruits = array(“apple”, “banana”, “orange”);
foreach ($fruits as $fruit) {
echo $fruit . “
“;
}
?>
// Using if-else statement
$age = 25;
if ($age >= 18) {
echo “You are an adult.”;
} else {
echo “You are not an adult.”;
}
?>
// Connecting to a MySQL database
$servername = “localhost”;
$username = “root”;
$password = “password”;
$dbname = “mydatabase”;
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die(“Connection failed: ” . $conn->connect_error);
}
echo “Connected successfully”;
?>
// Creating a session
session_start();
$_SESSION[‘username’] = “John”;
echo “Session created successfully”;
?>
// Sending email using PHP
$to = “[email protected]”;
$subject = “Hello”;
$message = “This is a test email.”;
$headers = “From: [email protected]”;
if (mail($to, $subject, $message, $headers)) {
echo “Email sent successfully.”;
} else {
echo “Email sending failed.”;
}
?>
// File handling in PHP
$filename = “example.txt”;
$file = fopen($filename, “w”);
if ($file) {
fwrite($file, “This is an example text.”);
fclose($file);
echo “File created and written successfully.”;
} else {
echo “Error in creating file.”;
}
?>
// Validating input using regular expressions
$email = “[email protected]”;
if (!preg_match(“/^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+.[a-zA-Z]{2,}$/”, $email)) {
echo “Invalid email.”;
} else {
echo “Valid email.”;
}
?>
// Generating a random password
$password = substr(md5(uniqid(mt_rand(), true)), 0, 8);
echo “Generated Password: ” . $password;
?>
// Encrypting and decrypting data
$data = “This is a secret message.”;
$key = “MySecretKey”;
$encrypted_data = openssl_encrypt($data, ‘AES-128-CBC’, $key, 0, ‘MyIV’);
$decrypted_data = openssl_decrypt($encrypted_data, ‘AES-128-CBC’, $key, 0, ‘MyIV’);
echo “Original Data: ” . $data . “
“;
echo “Encrypted Data: ” . $encrypted_data . “
“;
echo “Decrypted Data: ” . $decrypted_data . “
“;
?>
/* FAQ Section */
Q1. How can I run PHP code?
A1. To run PHP code, you need to have a web server such as Apache installed on your computer. You can create a new PHP file with a .php extension and place your code inside. You can then access the file through the web server using a browser.
Q2. What is the purpose of a session in PHP?
A2. A session in PHP allows you to store data that can be accessed across multiple pages. IT is commonly used for maintaining user login information, shopping carts, and user preferences.
Q3. How can I connect to a MySQL database in PHP?
A3. You can connect to a MySQL database in PHP using the mysqli or PDO extension. You need to provide the database credentials such as the servername, username, password, and database name. Once connected, you can perform various database operations like querying, inserting, updating, and deleting data.
Q4. How can I send an email using PHP?
A4. Sending an email using PHP can be done using the mail() function. You need to provide the recipient’s email address, subject, message, and optional headers. Make sure your web server is configured to send emails.
Q5. How can I validate user input using regular expressions in PHP?
A5. PHP provides the preg_match() function to validate user input using regular expressions. You can define a regular expression pattern to match against the user input and check if IT meets the desired format or criteria.
Q6. How can I generate a random password in PHP?
A6. PHP provides various functions for generating random passwords. One approach is to use the md5() and uniqid() functions to create a unique string and then truncate IT to the desired length.
Q7. How can I encrypt and decrypt data in PHP?
A7. PHP offers built-in functions for encrypting and decrypting data using various encryption algorithms. One commonly used encryption method is AES (Advanced Encryption Standard). The openssl_encrypt() and openssl_decrypt() functions can be used to perform encryption and decryption operations.
Q8. How can I handle files in PHP?
A8. PHP provides functions for handling files, such as fopen(), fwrite(), and fclose(). You can use these functions to create, read, write, and close files. Make sure you have proper file permissions to perform these operations.
Q9. How can I use if-else statements in PHP?
A9. IF-ELSE statements are used for conditional branching in PHP. You can check a condition using the if statement and execute different blocks of code based on whether the condition evaluates to true or false. The else statement allows you to define an alternative block of code to execute if the initial condition is false.
Q10. Can PHP be embedded within HTML code?
A10. Yes, PHP code can be embedded within HTML code using PHP tags . This allows you to dynamically generate HTML content based on the PHP code execution.