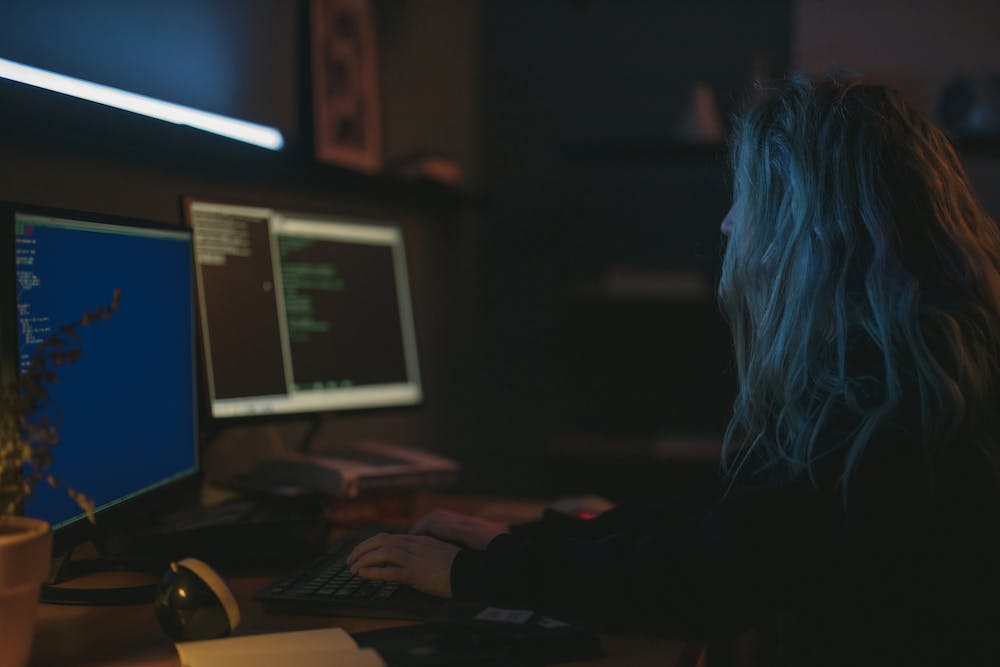
10 Essential JavaScript Concepts Every Developer Should Know
Introduction
JavaScript is an essential programming language for web development. IT enables developers to create interactive and dynamic web pages. Whether you’re a beginner or an experienced developer, IT‘s crucial to have a solid understanding of JavaScript concepts. In this article, we’ll explore ten essential JavaScript concepts that every developer should know to enhance their skills and build powerful web applications.
1. Variables
Variables are used to store data in JavaScript. They can hold various types of data, such as numbers, strings, or objects. To declare a variable, use the ‘let’ keyword. For example:
let myVariable = "Hello World";
2. Functions
Functions allow developers to encapsulate code and reuse IT whenever necessary. They take input, process IT, and return a value. In JavaScript, you can define functions using the ‘function’ keyword. Here’s an example:
function add(a, b) {
return a + b;
}
3. Conditional Statements
Conditional statements allow developers to make decisions based on certain conditions. The most common conditional statement is the ‘if’ statement. IT executes a block of code if a particular condition is true. Here’s an example:
let age = 18;
if (age >= 18) {
console.log("You are eligible to vote.");
}
4. Loops
Loops allow developers to repeat a block of code multiple times. The most commonly used loop in JavaScript is the ‘for’ loop. Here’s an example of using a ‘for’ loop to print numbers from 1 to 5:
for (let i = 1; i <= 5; i++) {
console.log(i);
}
5. Objects
Objects are essential in JavaScript as they allow developers to create complex data structures. An object consists of key-value pairs, where each key is a string (or symbol) and each value can be of any type. Here’s an example:
let person = {
name: "John",
age: 25,
address: "123 Street"
};
6. Arrays
Arrays are used to store multiple values in a single variable. In JavaScript, arrays are created using square brackets ‘[ ]’. Here’s an example:
let fruits = ["apple", "banana", "orange"];
7. DOM Manipulation
The Document Object Model (DOM) represents the web page structure and allows developers to manipulate its content. JavaScript provides powerful APIs to interact with the DOM, enabling dynamic updates to web pages. Here’s an example of changing the text content of an HTML element:
let element = document.getElementById("myElement");
element.textContent = "New content";
8. Event Handling
Event handling is crucial for creating interactive web applications. JavaScript allows developers to listen for certain events (such as a button click) and perform specific actions. Here’s an example of attaching an event listener to a button:
let button = document.getElementById("myButton");
button.addEventListener("click", function() {
console.log("Button clicked");
});
9. Error Handling
When developing JavaScript applications, IT‘s essential to handle errors effectively. JavaScript provides try-catch statements to handle and manage exceptions. Here’s an example:
try {
// Code that may throw an error
} catch (error) {
console.log("An error occurred: " + error.message);
}
10. Modules
Modules allow developers to organize and split their code into separate files, improving reusability and maintainability. With modules, developers can export and import functions, objects, or variables between different files. Here’s an example:
// utils.js
export function multiply(a, b) {
return a * b;
}
// main.js
import { multiply } from "./utils.js";
console.log(multiply(5, 2)); // Output: 10
Conclusion
Mastering these ten essential JavaScript concepts will significantly improve your skills as a web developer. Variables, functions, conditional statements, loops, objects, arrays, DOM manipulation, event handling, error handling, and modules are fundamental concepts that form the backbone of JavaScript programming. Continuously practicing and building projects using these concepts will help you become a proficient JavaScript developer.
FAQs
1. What is JavaScript?
JavaScript is a popular programming language used to create interactive and dynamic web pages.
2. Can I declare a variable without using the ‘let’ keyword?
Yes, in older versions of JavaScript, you could declare a variable using the ‘var’ keyword. However, IT‘s recommended to use ‘let’ to declare variables in modern JavaScript.
3. How can I access an element in the DOM using JavaScript?
You can access elements in the DOM using methods like ‘getElementById’, ‘getElementsByClassName’, or ‘querySelector’.
4. What are the benefits of using modules in JavaScript?
Modules help organize code, improve reusability, and make IT easier to maintain large-scale JavaScript applications.
5. How can I handle errors in JavaScript?
You can use a try-catch statement to catch and handle errors in JavaScript code.
In conclusion, understanding these ten essential JavaScript concepts is paramount for any developer looking to excel in web development. By mastering variables, functions, conditional statements, loops, objects, arrays, DOM manipulation, event handling, error handling, and modules, developers can build robust and interactive web applications efficiently. Continual practice and implementation of these concepts in real-world projects will further enhance your JavaScript skills. So, start exploring and experimenting with JavaScript to empower your web development journey.