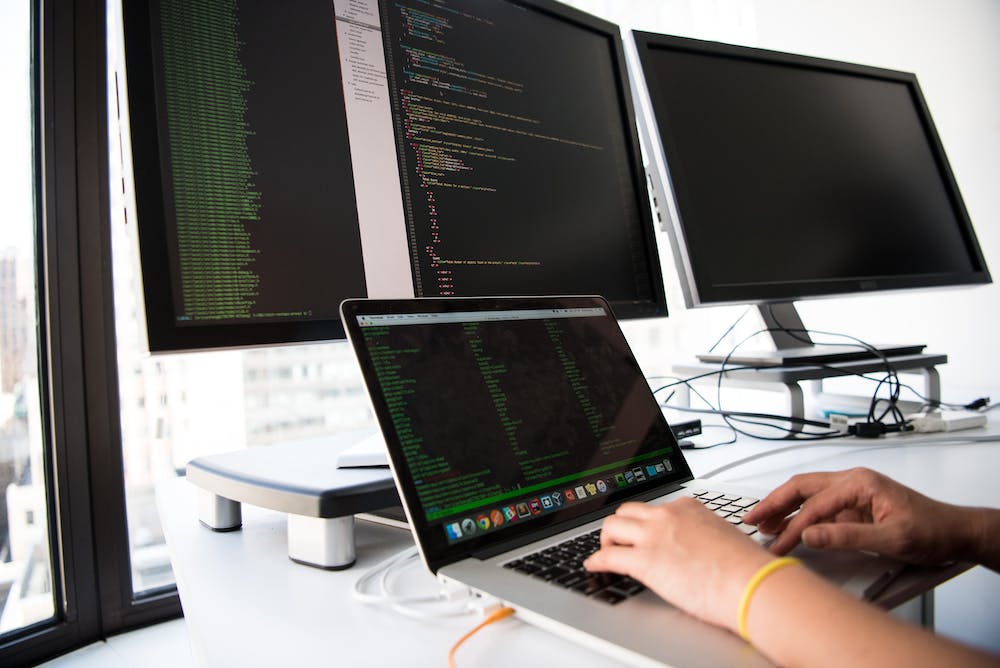
10 Essential JavaScript Code Examples for Beginners
JavaScript is a powerful programming language that is widely used for web development. IT allows you to add interactivity and dynamism to your websites. For beginners, mastering JavaScript can be a bit challenging. However, with practice and exposure to real-world examples, you can quickly grasp the concepts and become proficient in writing JavaScript code. In this article, we will explore 10 essential JavaScript code examples that are suitable for beginners.
1. Hello World!
The classic “Hello World!” example is the perfect starting point for any programming language. To display this message in JavaScript, use the following code:
// Output "Hello World!"
console.log("Hello World!");
2. Variables and Data Types
Variables are essential in programming. They allow us to store and manipulate data. In JavaScript, you can declare variables using the var
keyword:
// Declare a variable and assign a value
var age = 25;
// Output the value of the variable
console.log(age); // Output: 25
3. Operators and Expressions
Operators are used to perform operations on variables and values. Here’s an example of using arithmetic operators:
var x = 5;
var y = 3;
var sum = x + y;
console.log(sum); // Output: 8
4. Conditional Statements
Conditional statements allow you to execute specific code blocks based on certain conditions. For example, using an if statement to determine if a number is even or odd:
var num = 6;
if (num % 2 === 0) {
console.log("Even");
} else {
console.log("Odd");
}
5. Loops
Loops are used to repeatedly execute a block of code. The most commonly used loop in JavaScript is the for
loop:
for (var i = 0; i < 5; i++) {
console.log(i);
}
6. Functions
Functions allow you to group and reuse blocks of code. Here’s an example of a function that calculates the area of a rectangle:
function calculateArea(length, width) {
var area = length * width;
return area;
}
var rectangleArea = calculateArea(5, 3);
console.log(rectangleArea); // Output: 15
7. Arrays
Arrays are used to store multiple values in a single variable. Here’s an example of an array that stores a list of fruits:
var fruits = ["apple", "banana", "orange"];
console.log(fruits); // Output: ["apple", "banana", "orange"]
8. Objects
Objects allow you to store key-value pairs. They are useful for representing real-world entities. Here’s an example of an object that represents a person:
var person = {
name: "John",
age: 25,
profession: "Developer"
};
console.log(person.name); // Output: "John"
9. Document Object Model (DOM) Manipulation
The DOM is a programming interface for HTML and XML documents. JavaScript can be used to manipulate the DOM and change the content of web pages. Here’s an example of changing the text of an element with the id “myElement”:
// HTML: <p id="myElement">Old Text</p>
var element = document.getElementById("myElement");
element.innerHTML = "New Text";
10. Event Handling
JavaScript can handle various events such as button clicks, mouse movements, and keyboard inputs. Here’s an example of adding an event listener to a button:
// HTML: <button id="myButton">Click Me</button>
var button = document.getElementById("myButton");
button.addEventListener("click", function() {
alert("Button Clicked!");
});
Frequently Asked Questions (FAQs)
Q: Is JavaScript case-sensitive?
A: Yes, JavaScript is case-sensitive. For example, variable
and Variable
are treated as different variables.
Q: Can I use JavaScript to validate user input on a form?
A: Yes, JavaScript can be used to validate and process user input on a form before IT is submitted to a server.
Q: Do I need to download or install JavaScript to use IT?
A: No, JavaScript is natively supported by modern web browsers, so you don’t need to install anything separately.
Q: Can I use JavaScript for backend development?
A: Yes, JavaScript can be used for both frontend (client-side) and backend (server-side) development. Node.js is a popular runtime that allows JavaScript to be executed on the server.
Q: Is IT necessary to learn HTML and CSS before learning JavaScript?
A: While IT is not mandatory, having a basic understanding of HTML and CSS will greatly enhance your ability to work with JavaScript, as JavaScript is often used to interact with HTML and manipulate CSS styles.
Q: Are there any resources or tutorials available to learn more about JavaScript?
A: Yes, there are countless online resources, tutorials, and documentation available to learn JavaScript. Some popular platforms include Mozilla Developer Network (MDN), W3Schools, and freeCodeCamp.