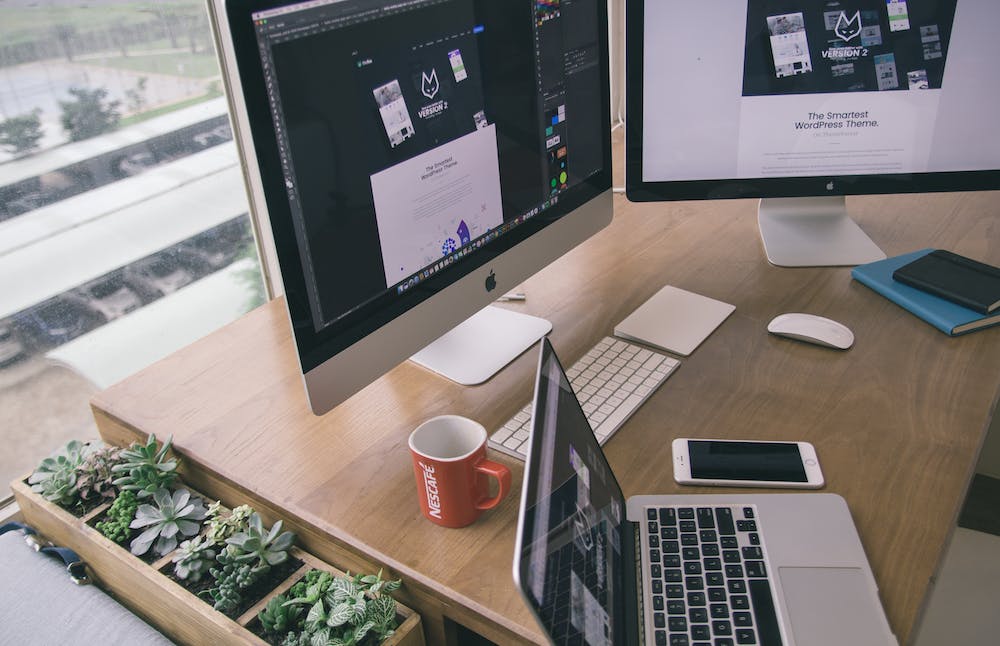
Python, as a programming language, has gained immense popularity in recent years. IT offers an easy-to-understand syntax and a wide range of libraries and frameworks, making IT suitable for beginners and experienced programmers alike. With Python, you can develop powerful programs and scripts that can simplify complex tasks and improve your coding skills.
In this article, we will explore ten easy Python programs that are perfect for beginners but can also enhance the skills of experienced developers. Let’s dive in!
1. Hello, World!
No programming article would be complete without the traditional “Hello, World!” program. This simple Python program prints the famous phrase that marks the beginning of every programmer’s journey.
“`python
print(“Hello, World!”)
“`
2. Calculator
A calculator program is an excellent way to practice fundamental arithmetic operations in Python. IT enables you to perform addition, subtraction, multiplication, and division, empowering you to solve complex equations.
“`python
num1 = float(input(“Enter the first number: “))
num2 = float(input(“Enter the second number: “))
print(“Sum:”, num1 + num2)
print(“Difference:”, num1 – num2)
print(“Product:”, num1 * num2)
print(“Quotient:”, num1 / num2)
“`
3. Guess the Number
This program allows the user to guess a randomly generated number within a given range. IT enhances your logic and conditional statement skills while providing a fun and interactive experience.
“`python
import random
number_to_guess = random.randint(1, 100)
guess = int(input(“Guess a number between 1 and 100: “))
while guess != number_to_guess:
if guess < number_to_guess:
print(“Too low!”)
else:
print(“Too high!”)
guess = int(input(“Guess again: “))
print(“Congratulations! You guessed the number correctly!”)
“`
4. Tic-Tac-Toe
Implementing a Tic-Tac-Toe game is an exciting project that helps you understand concepts like nested lists, conditional statements, and loops. You can challenge yourself by adding features like player vs. computer functionality.
“`python
def display_board(board):
for row in board:
print(“|”.join(row))
board = [[” “, ” “, ” “], [” “, ” “, ” “], [” “, ” “, ” “]]
display_board(board)
“`
5. Palindrome Checker
A palindrome is a word, phrase, number, or sequence that reads the same backward as forward. By writing a program that checks if a given string is a palindrome, you can develop your string manipulation and conditional statement skills in Python.
“`python
def is_palindrome(word):
reversed_word = word[::-1]
if word == reversed_word:
return True
else:
return False
print(is_palindrome(“racecar”))
“`
6. Fibonacci Sequence
The Fibonacci sequence is a series of numbers in which each number is the sum of the two preceding ones. Implementing a program that generates the Fibonacci sequence enhances your loop and mathematical calculation abilities.
“`python
def fibonacci_sequence(n):
sequence = [0, 1]
for i in range(2, n):
sequence.append(sequence[i-1] + sequence[i-2])
return sequence
print(fibonacci_sequence(10))
“`
7. Countdown Timer
A countdown timer is useful for various applications, including games and productivity tools. By creating a countdown timer program, you can practice working with time-related functions and developing interactive real-time applications.
“`python
import time
def countdown_timer(seconds):
for i in range(seconds, 0, -1):
print(i)
time.sleep(1)
print(“Time’s up!”)
countdown_timer(10)
“`
8. File Renamer
The file renamer program allows you to rename multiple files in a directory simultaneously. This project enables you to manipulate file systems and utilize Python’s file handling capabilities.
“`python
import os
directory = “path/to/directory”
for filename in os.listdir(directory):
file_extension = os.path.splitext(filename)[-1]
new_filename = “new” + file_extension
os.rename(os.path.join(directory, filename), os.path.join(directory, new_filename))
“`
9. Weather Forecast
Integrating an API to fetch weather data and displaying IT as a forecast is a valuable project. IT enables you to work with APIs, handle JSON data, and develop programs that provide real-time information.
“`python
import requests
import json
def weather_forecast(city):
response = requests.get(f”https://api.weatherapi.com/v1/current.json?key=YOUR_API_KEY&q={city}”)
data = json.loads(response.text)
return data[“current”][“condition”][“text”]
print(weather_forecast(“New York”))
“`
10. URL Shortener
A URL shortener is a great project to learn about handling user inputs, string manipulation, and utilizing external libraries. IT allows you to create shortened versions of long URLs, improving their shareability.
“`python
from pyshorteners import Shortener
def shorten_url(url):
shortener = Shortener()
shortened_url = shortener.tinyurl.short(url)
return shortened_url
print(shorten_url(“https://www.example.com/this-is-a-long-link”))
“`
Conclusion
These ten easy Python programs provide a wide range of opportunities for programmers to practice their skills while creating useful and fun projects. By working on these programs, you can gain hands-on experience with important Python concepts and functionalities such as string manipulation, conditional statements, loops, and API integration.
Each program showcased in this article serves a specific purpose and helps you improve your programming abilities step by step. Whether you are a beginner looking to enhance your skills or an experienced developer seeking to reinforce your knowledge, these programs will undoubtedly revolutionize your coding abilities and deepen your understanding of Python.
FAQs
Q: How can I run these Python programs on my computer?
A: To run these Python programs, start by installing Python on your computer. You can download the latest version of Python from the Python official Website. Then, save the program code in a file with the “.py” extension. Open a terminal or command prompt, navigate to the directory containing the program file, and use the command “python filename.py” to execute the program.
Q: Can I modify these programs and add new features to them?
A: Absolutely! These programs provide a foundation upon which you can build and expand. Feel free to modify the code, experiment with new features, and add your personal touch. Programming is all about creativity, innovation, and customization.